Overview
Mod Manager is a desktop application that assists NUS students in managing tasks, schedules, and contacts for their modules in a semester. Our team, which consists of five software developers, took over an existing desktop Java application Address Book (Level 3) with about 6 KLoC and evolve it into our Mod Manager with more than 10 KLoC. The project spans over a period of eight weeks, where each of us idealise and design the product, utilise CI/CD for weekly project enhancements, implement features and functionality, write documentation (User Guide & Developer Guide), and take part in Quality Assurance.
Technologies: Java, JavaFx, GitHub, Intellij IDEA
The following sections document all the contributions that I have made to Mod Manager.
Summary of contributions
-
Code contributed: I personally contributed almost 4 KLoC to Mod Manager. All my code contributions can be found here.
-
Major enhancement: idealise and design the
Task
component of Mod Manager. TheTask
component allows NUS students to manage their tasks, such as programming assignments, homework, tutorials, reviewing lecture content, exam revision for their respective modules in a semester. Implement most of the commands available for theTask
component of Mod Manager.-
What it does: every
Task
has a description, a time frame (for example,13/04/2020 23:59
), and aModule
it belongs to. It allows users to organise and manage their tasks easier, with commands such as CRUD, mark the task as completed, view uncompleted tasks, view tasks byModule
, findTask
by its description, and search for aTask
by its date, month, or year. -
Justification: This feature improves the product significantly because every NUS student has a lot of things to do in the semester, and
Task
management offers a way for them to plan, and manage their tasks better. TheTask
component blends in well withMod Manager
and its other components, for example, everyTask
is allocated to an academicModule
. -
Highlights: The implementation of the project is tedious. It required a great amount of effort to understand the original AddressBook (Level 3) code given in order to morph and build upon this original project. Every small increment in the
Task
features require changes to multiple existing parts of the code, which requires using debugging tools and tracing the code to understand the code execution sequence. For example, with a small change in theTask
class design, multipleJUnit
test cases and dataStorage
design need to be changed. TheTask
component also has some dependencies on other components, as well as multiple other components have dependencies on theTask
component. This requires good communication skills between the team to notify each other every time the high-level design ofTask
or other components change, even with just a slight change. TheTask
component also required an in-depth analysis of design alternatives, which lead to incremental changes over the period of the project. For the commands implemented, input validation and data formatting is critical to avoid unexpected behaviours to our application. -
Credits: The idea is adapted from AddressBook (Level 3) and the Duke project. Huge thanks to my teammates who have gave feedback and improved on the design of the
Task
, which includes making it more OOP to integrate it better with the rest of the application, and adding thetaskId
feature to support the identification of tasks in ourTask
commands.
-
-
Minor enhancement: design and implement the UI to represent a
Task
. The design is useful for the value proposition of the product, as it helps you to recognise what tasks are completed and what are not easily.
Dark red color indicates a task that is not yet done.

Green color indicates a task that is already completed.

-
Other contributions:
-
Project management: mainly responsible for managing deadlines and deliverables for our team.
-
Ensure that all milestone progress are reached (which includes team-based and individual-based tasks). We managed to achieve more than 90% of the recommended milestone tasks, much higher than the minimum requirement of 60% of the class.
-
Maintained the issue tracker and milestones for team repo
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
Contributions to the User Guide
Given below are some sections I contributed to the User Guide.
They comprise some of the commands for the |
For your easier understanding of the content below, every Task in a Module has an ID ,
which uniquely identifies the task in the module. The ID is important for us to differentiate a task
from the others in the Module , since two Task s may have the same description and time frame.
The Task component uses Singapore’s standard date format (dd/MM/yyyy ).
|
Marking a task as done (Bao)
You can mark the task as done in the module in Mod Manager.
A newly added task will be not done by default. |
Editing a task will not change the done/not done status of the task. |
Tasks that are already marked as done cannot be re-marked as done. |
Format:
-
task done /code MOD_CODE /id ID_NUMBER
Command properties:
Example:
You can mark a task as done in the module. To mark the task with
task ID ID_NUMBER
in module MOD_CODE
to be done, you can type in the following command:
task done /code CS2105 /id 224
and hit Enter
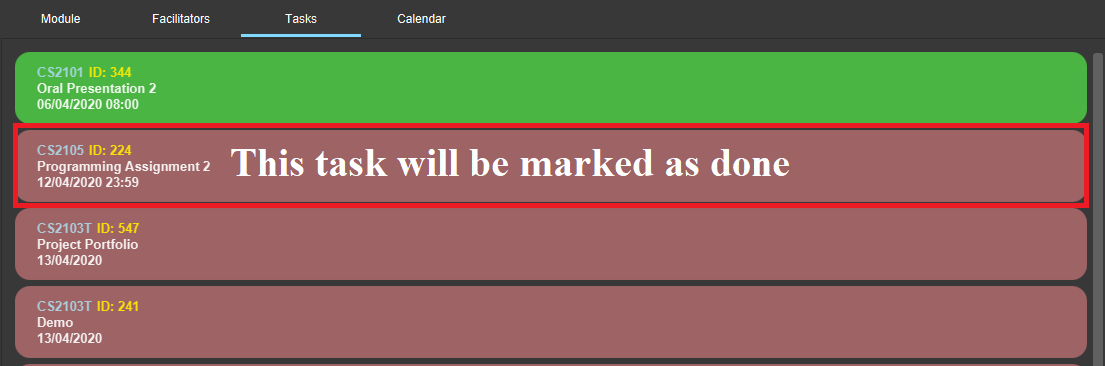
task done /code CS2105 /id 224
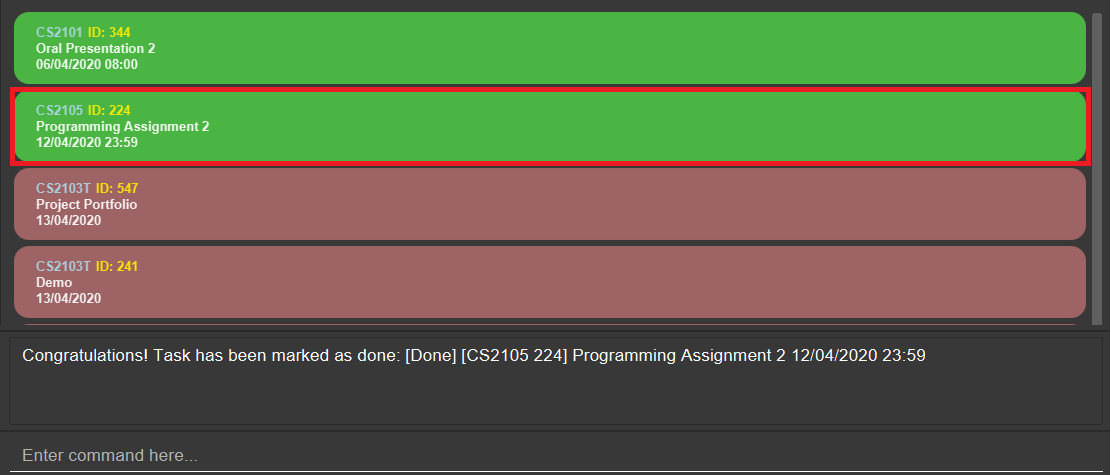
task done /code CS2105 /id 224
The task card has changed to green; which means our task has been marked as done. Hooray! We just completed a task.
Finding tasks by description (Bao)
You are browsing through the task list. But there are too many tasks! You suddenly remember a specific task that you want to do, but you can only vaguely remember its description, e.g. something related to assignment.
This command is exactly what you need. In your case, you can find all tasks that
contain the word assignment
, which may include Programming Assignment
,
written assignment
, Take-home Lab Assignment
(note that it can be case-insensitive).
If you remember multiple words in your wanted tasks, you may also type in multiple words as you want.
Tasks that meet at least one of the keywords you provided will be shown to you.
Format:
-
task find DESCRIPTION [MORE_DESCRIPTIONS]…
Command properties:
Examples:
To find tasks that contain the word oral
, assign
, or tut
in their description,
you can type in the following command:
task find oral assign tut
and press Enter
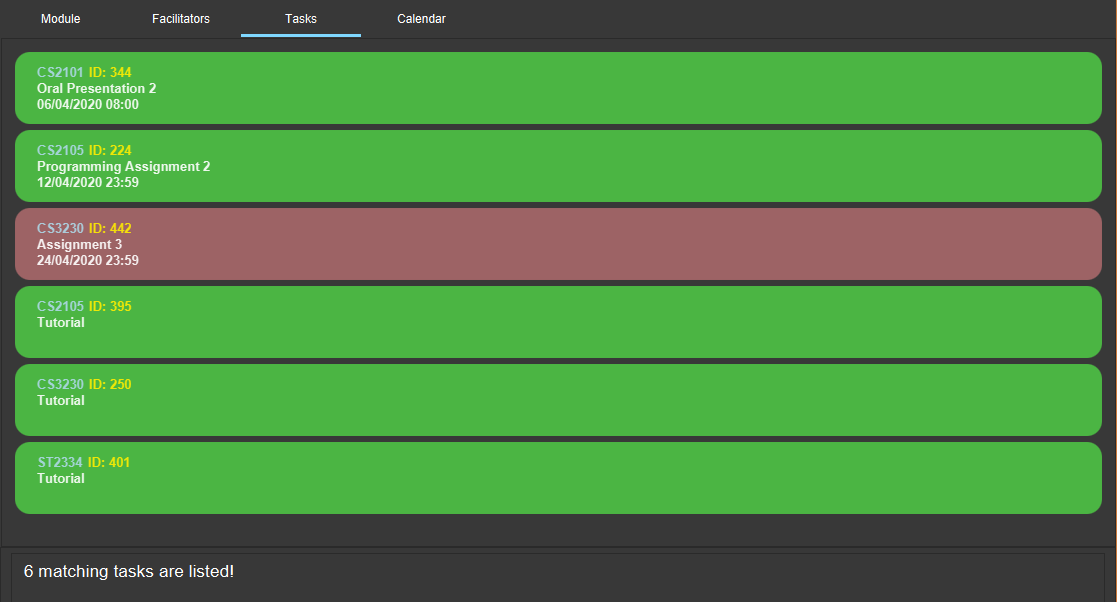
task find oral assign tut
, all matching tasks are displayedExplanation:
- Oral Presentation 2
contains Oral
which matches oral
(case-insensitive).
- Programming Assignment 2
contains Assignment
which matches assign
(case-insensitive, and words can be partial match)
- Similarly, Assignment 3
will match assign
, and Tutorial
will match tut
- As long as a task’s description matches one of the keywords provided, it will be shown.
You can try typing in task find assign tut oral
and press Enter. This will return the same list
of tasks, since the ordering of the keywords does not matter.
Other examples:
-
task find homework
Finds all tasks that contain the wordhomework
in their description -
task find math coding
Finds all tasks that contain the wordmath
orcoding
in their description
Contributions to the Developer Guide
I idealised and designed the Given below are some sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project._ |
Implementation, Task Component
Marking a task as done (Bao)
The marking a task as done command allows users to mark a certain Task
in a Module
as done,
based on its task ID called taskNum
.
This feature is facilitated by TaskCommandParser
, TaskMarkAsDoneCommandParser
and TaskMarkAsDoneCommand
.
The operation is exposed in the Model
interface as Model#setTask()
.
Given below is an example usage scenario and how the marking task as done mechanism behaves at each step.
-
The user executes the task mark as done command and provides the
moduleCode
and thetaskNum
of the task to be marked as done. -
TaskMarkAsDoneCommandParser
creates a newTaskMarkAsDoneCommand
based on themoduleCode
andtaskNum
. -
LogicManager
executes theTaskMarkAsDoneCommand
. -
TaskMarkAsDoneCommand
retrieves themoduleCode
andtaskNum
of the task to be marked as done, and then retrieves the currently existingTask
fromModManager
. -
TaskMarkAsDoneCommand
creates a clone of the retrievedTask
, then mark this newTask
as done. -
ModManager
sets the existing task to the new task, marked as done in theUniqueTaskList
. -
ModelManager
updates thefilteredTasks
inModelManager
.
The following sequence diagram shows how the task mark as done command works:
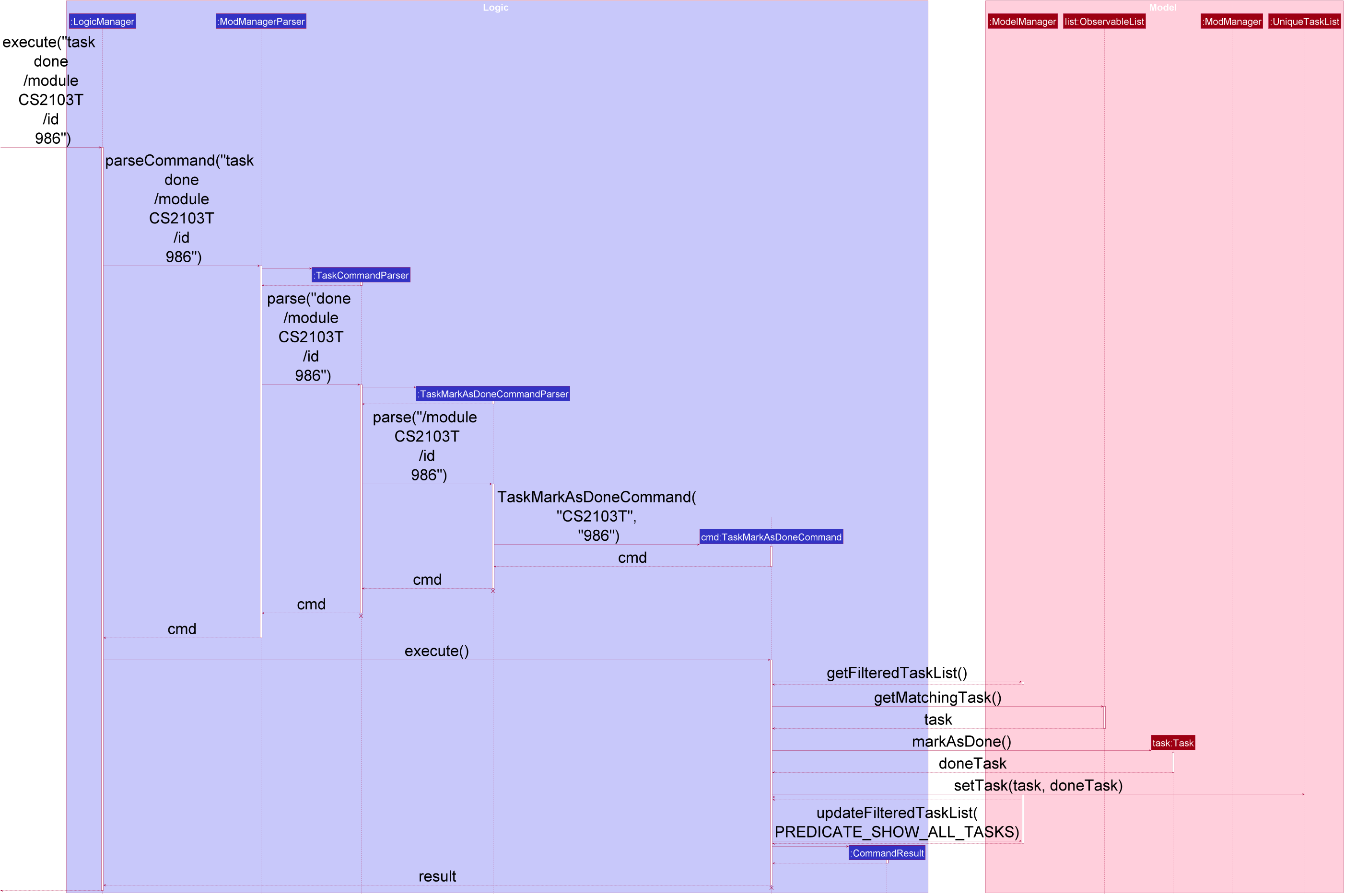
task done /code CS2103T /id 986
Command
The lifeline for TaskCommandParser , TaskMarkAsDoneCommandParser , and TaskMarkAsDoneCommand should end at
the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of the diagram.
|
The following activity diagram summarizes what happens when a user executes the task mark as done command:
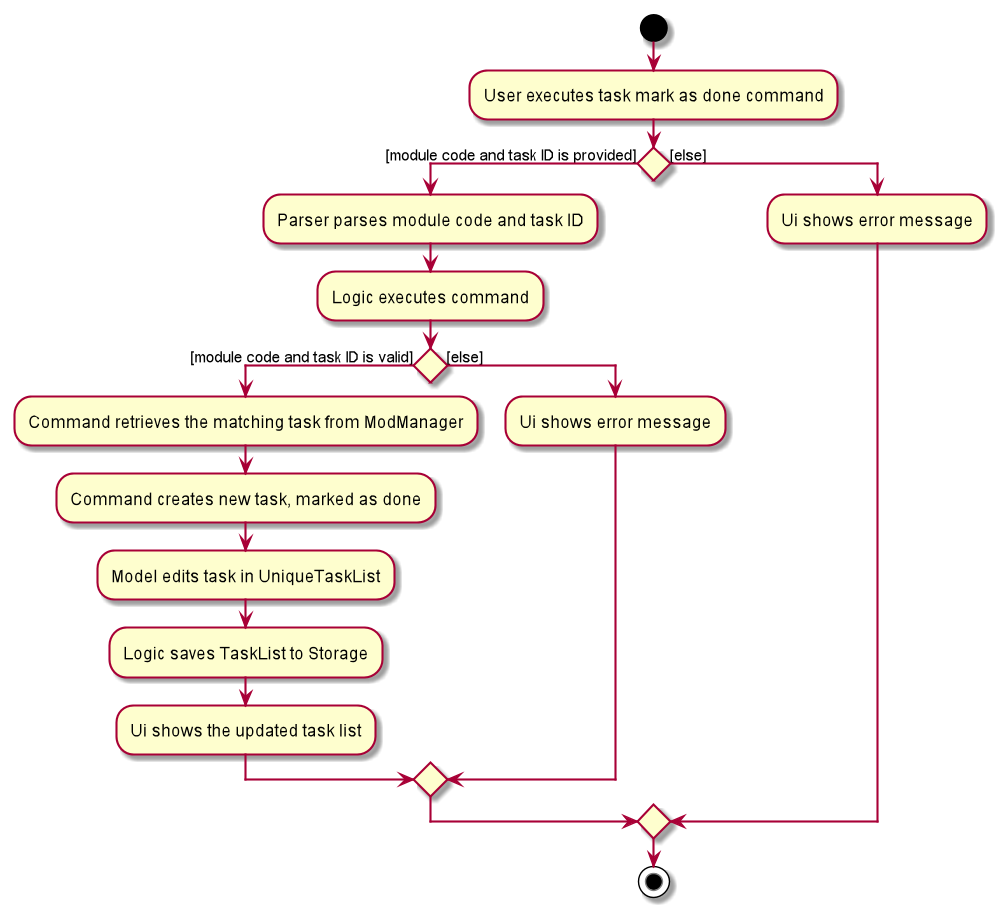
task done
CommandFinding tasks by description (Bao)
The find task feature allows users to find a task by its description in Mod Manager.
This feature is facilitated by TaskCommandParser
, TaskFindCommandParser
and TaskFindCommand
.
The operation is exposed in the Model
interface as Model#updateFilteredTaskList()
.
Given below is an example usage scenario and how the task find mechanism behaves at each step:
-
The user executes the task find command and provides the descriptions of the tasks to search for.
-
TaskFindCommandParser
creates a newTaskFindCommand
based on the descriptions. -
LogicManager
executes theTaskFindCommand
. -
ModelManager
updates thefilteredTasks
inModelManager
.
The following sequence diagram shows how the task find command works:
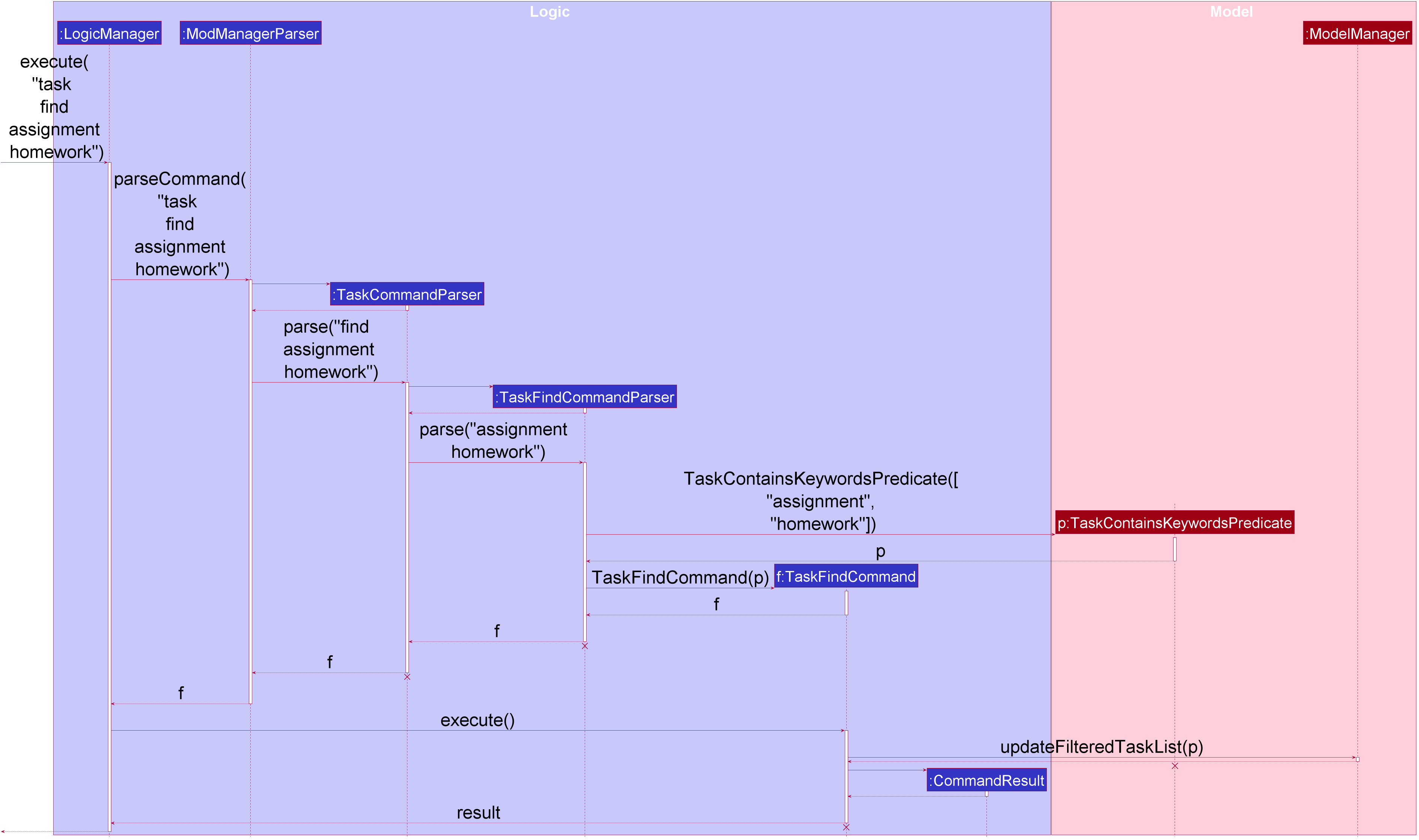
task find assignment homework
Command
The lifeline for TaskCommandParser , TaskFindCommandParser , TaskFindCommand and TaskContainsKeywordsPredicate should end at
the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of the diagram.
|
The following activity diagram summarizes what happens when a user executes a task find command:
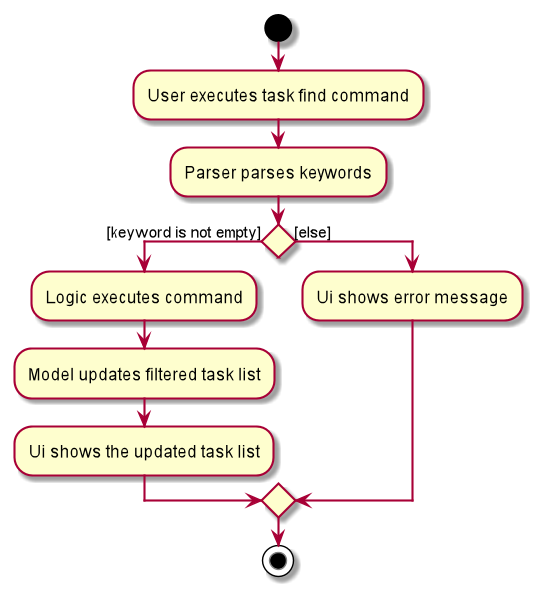
task find
Command