Overview
For this software engineering project, my team and I were tasked with morphing Address Book Level 3 (AB3) application into a better and different product. We decided to make ModManager, which aims to help students in managing module related information such as modules, classes, tasks and facilitators. Mod Manager is made for those who prefer to work with a Command Line Interface (CLI) while still having the benefits of a Graphical User Interface (GUI). It is written in Java and has about 25kLoC.
Below is an example of what ModManager looks like:
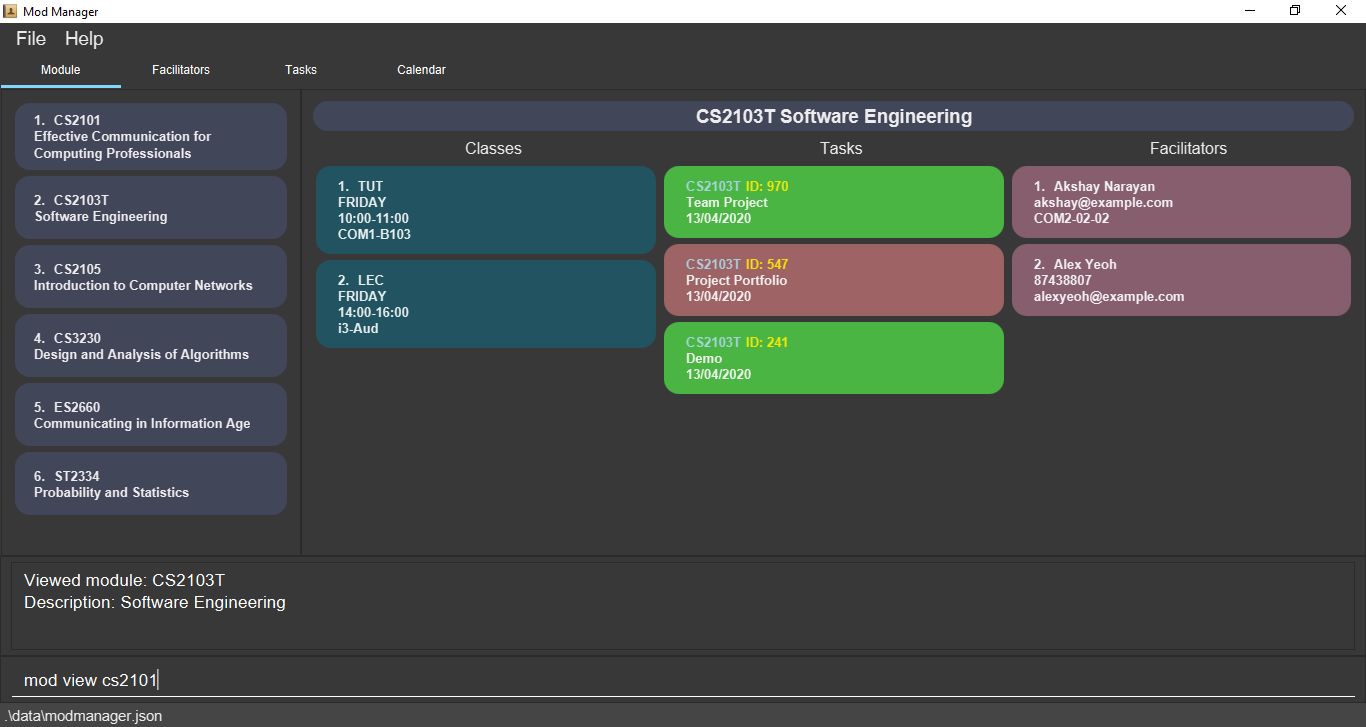
Summary of contributions
This section summarizes my contribution to ModManager.
Major enhancement:
-
Implemented the class management feature
-
What it does: allows the user to add, view, edit and delete classes.
-
Justification: This feature improves the product because a user can manage their classes and find upcoming class, which allow the user to better prioritize their time and prepare for their classes.
-
Highlights: There is some dependency between this feature and other features. It required an in-depth analysis of design alternatives. The implementation was tough due to parsing. Since there were various arguments and command formats considered, parsing was tougher than usual.
-
Minor enhancement:
-
Sorted the classes by time.
-
Enhanced the aesthetics of the GUI.
Code contributed:
Other contributions:
-
Project management:
-
Merged pull requests
-
-
Enhancements to existing features:
-
Documentation:
-
Community:
-
PRs reviewed (with non-trivial review comments) : #95
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Class feature : class
You can add, find, edit or delete classes within Mod Manager.
When managing your classes, you should take note of the following areas in the main viewing area. To find the main viewing area, you can refer to section 3.1.
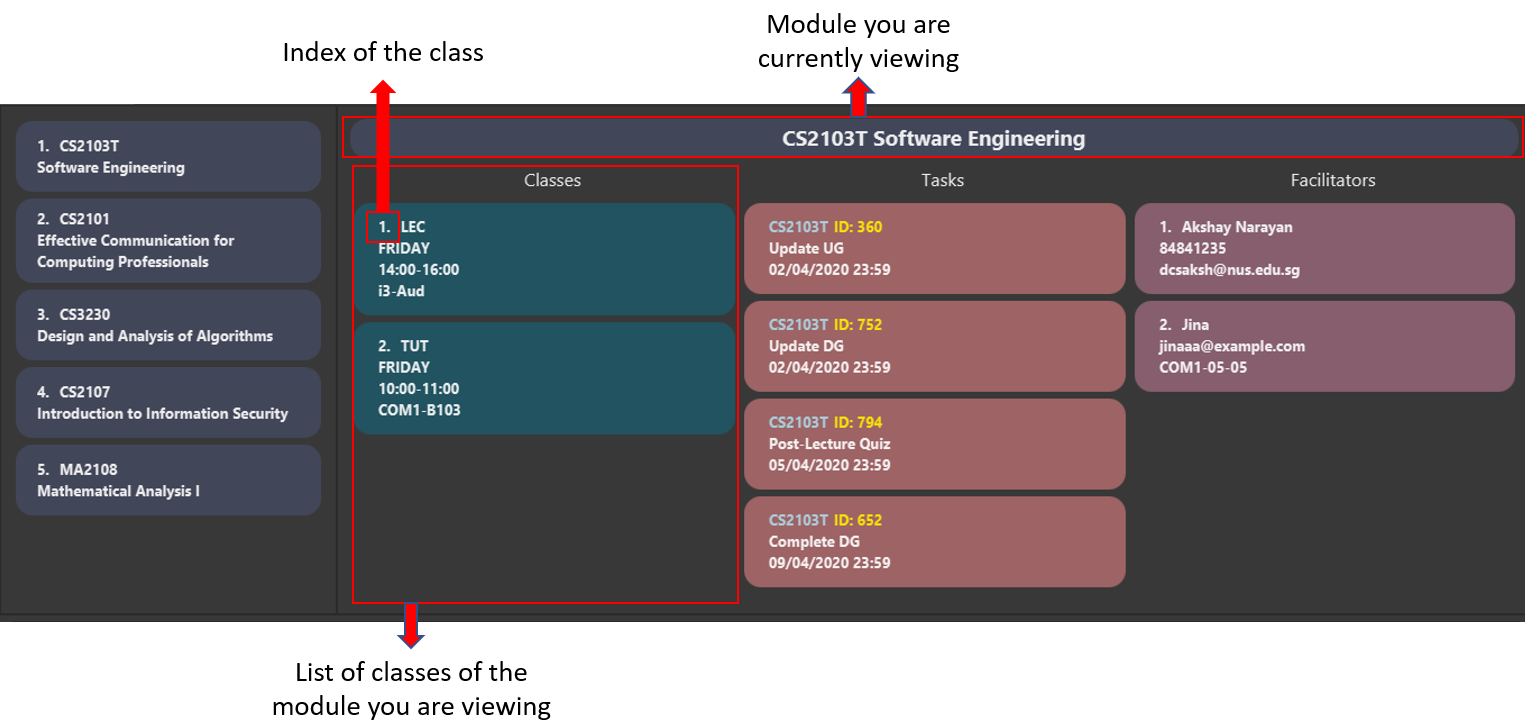
Adding a class (Heidi)
You can add a class to a module.
Format:
-
class add /code MOD_CODE /type CLASS_TYPE /at DAY START_TIME END_TIME [/venue VENUE]
Command properties:
Example:
You have a CS2103T lecture on Friday from 14:00 to 16:00 at i3-Aud. Before adding that class, Mod Manager looks like what you see in the figure below.
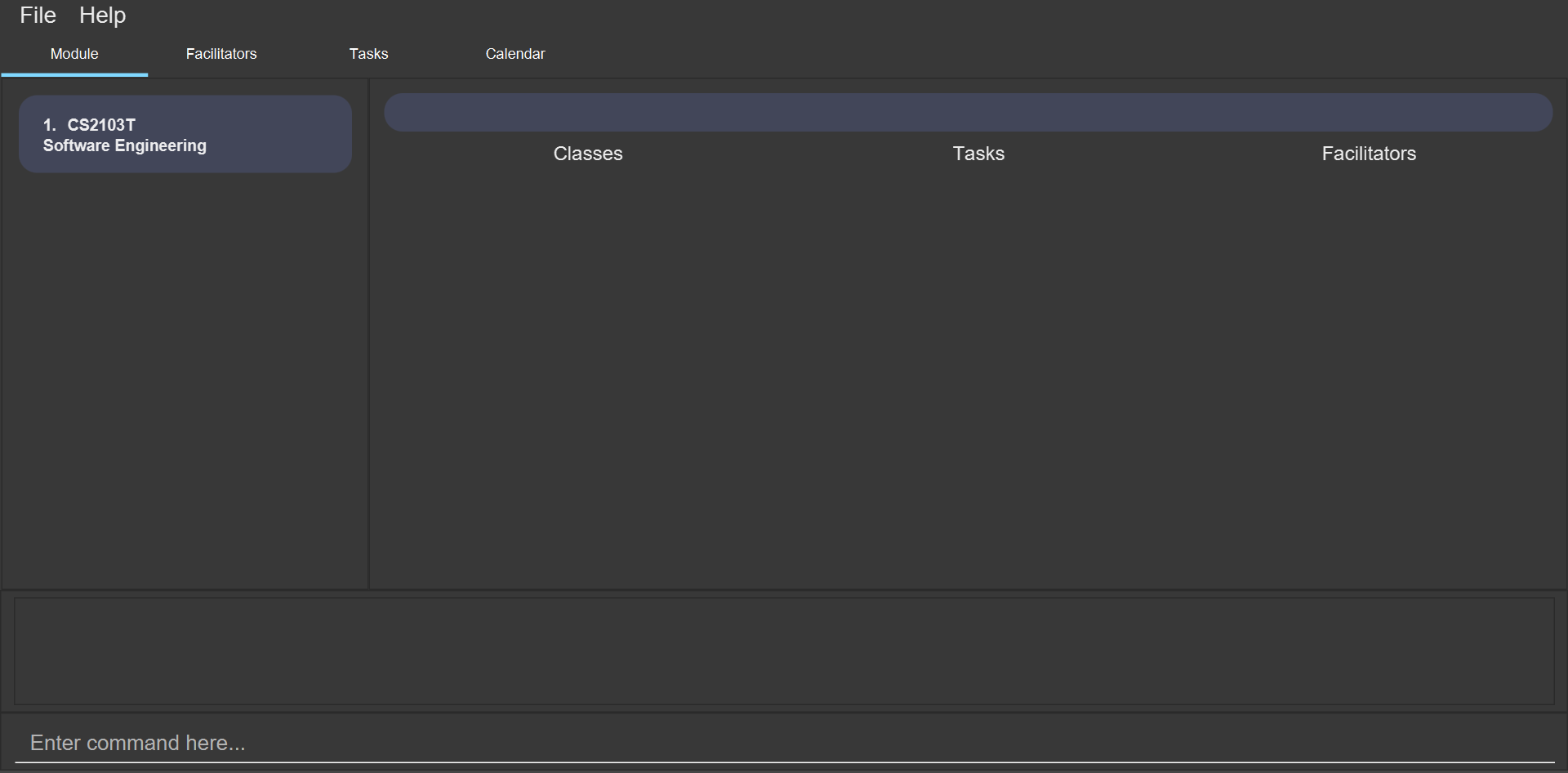
To add that class, you can type:
class add /code CS2103T /type LEC /at FRIDAY 14:00 16:00 /venue i3-Aud
.
You will see a new class added to the module CS2103T as shown in the figure below.
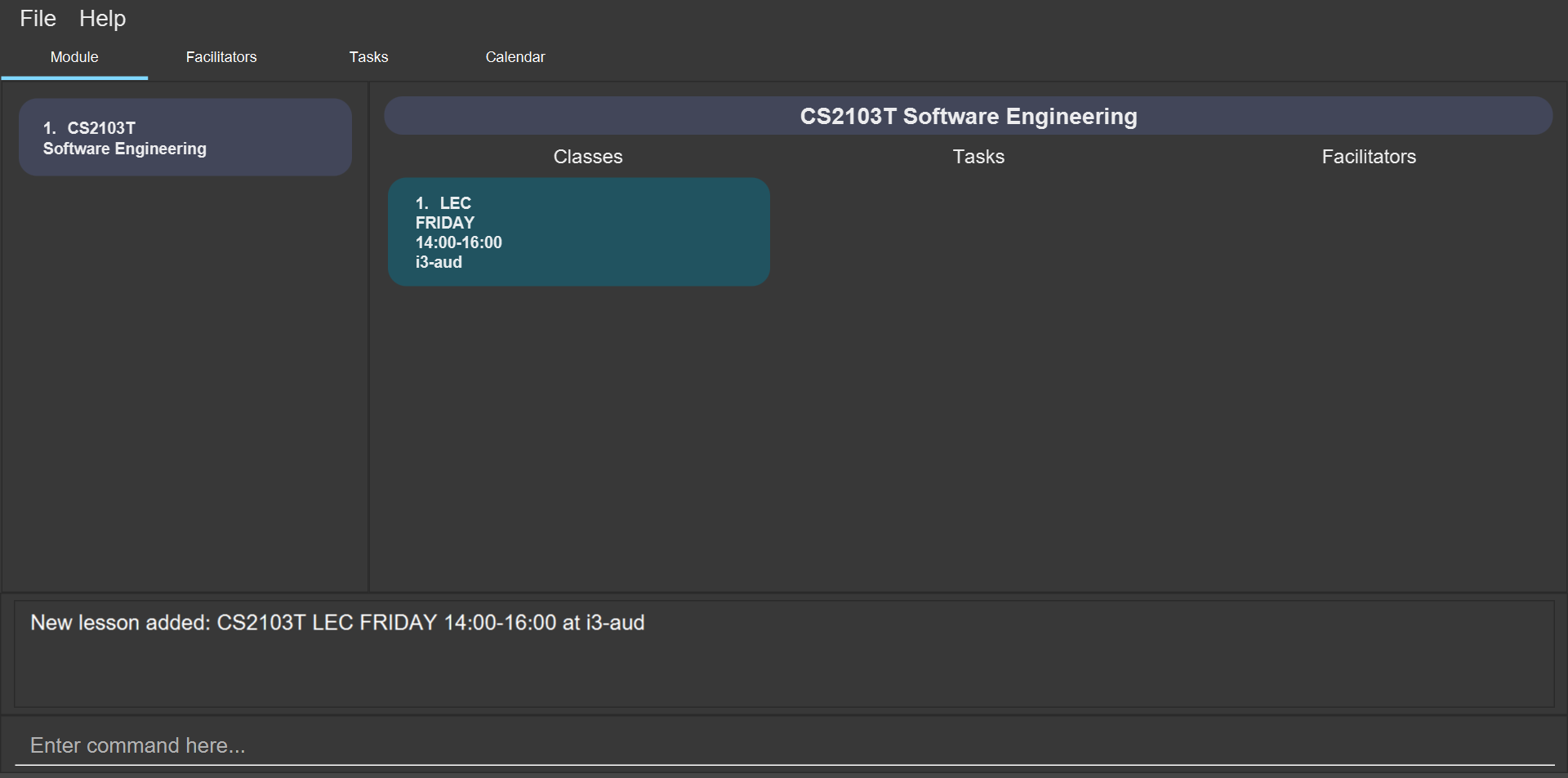
Finding classes by day (Heidi)
You can find classes occurring on a particular day.
Format:
-
class find /at DAY
Command properties:
Example:
To know what classes you have on Friday, you can type class find /at friday
and you will be able to see the classes in the result display box as shown in the figure below.
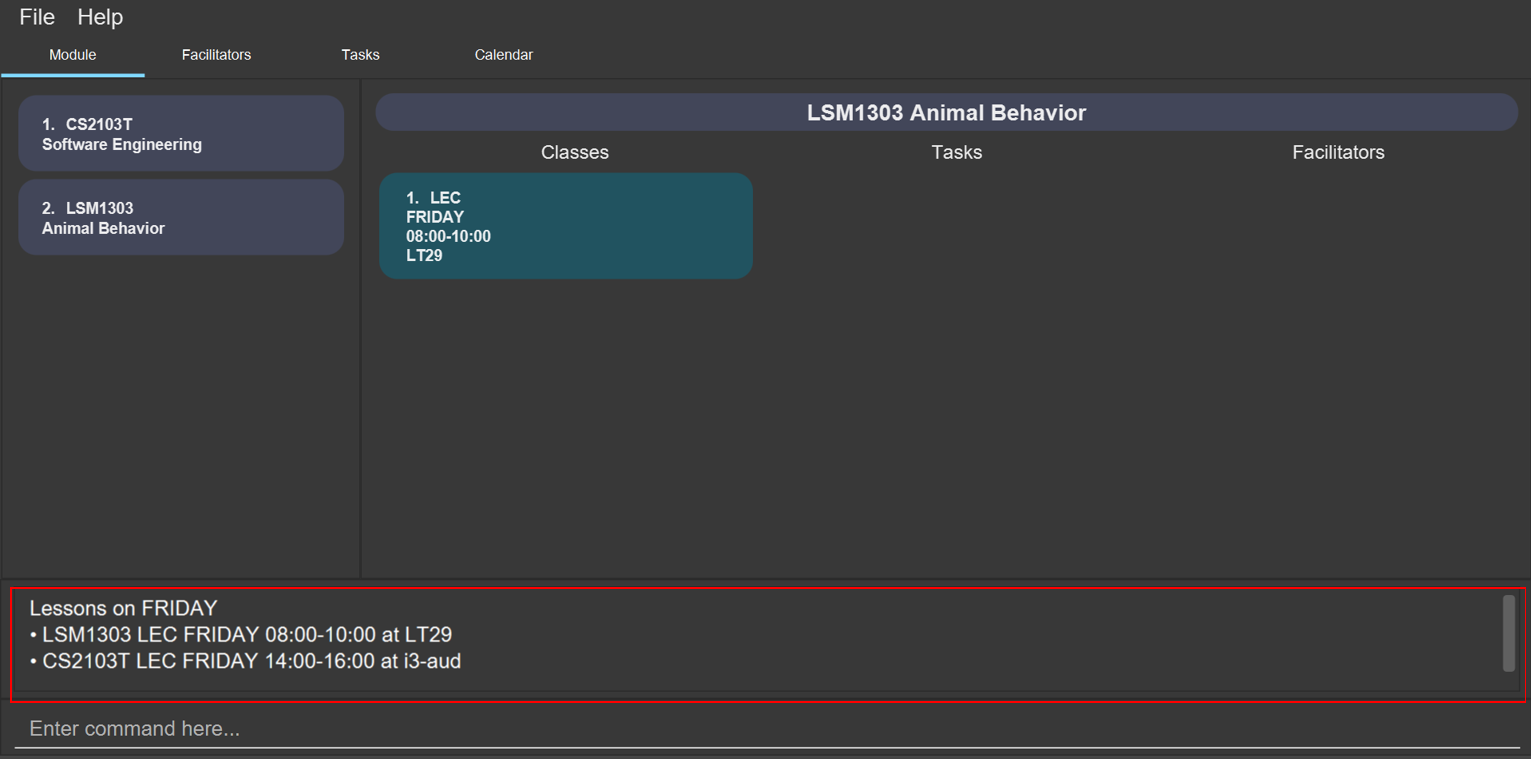
Finding next class (Heidi)
You can find the next class that will start soon.
Format:
-
class find /next
Example:
After typing class find /next
, you will be directed to the module’s page and you will see the class as shown in the figure below.
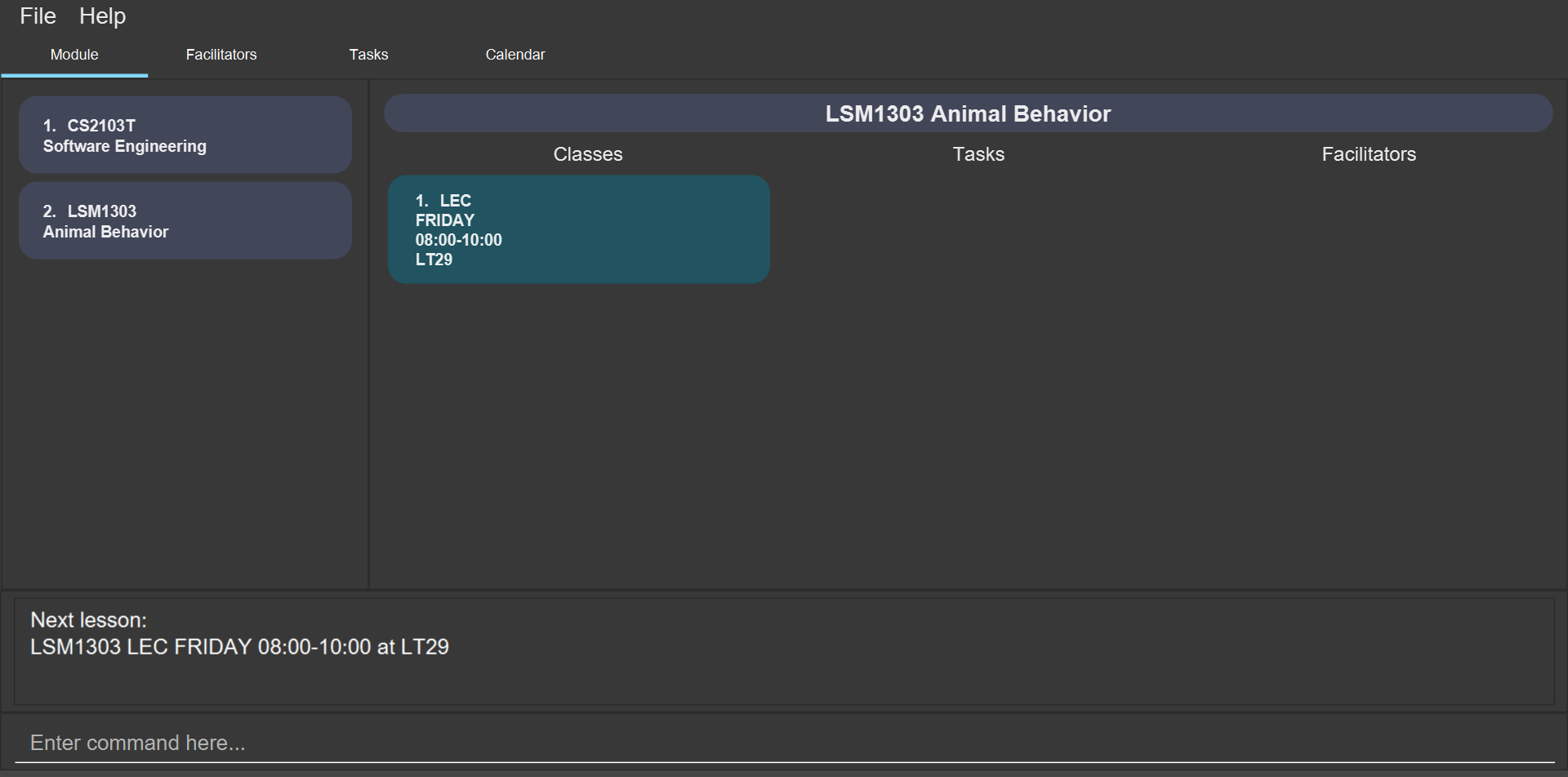
Editing a class (Heidi)
You can edit the information of the class. The class to be edited is selected by its index in the displayed module’s class list. You can view the module’s class list by using mod view MOD_CODE
as stated in section 3.2.3.
Format:
-
class edit INDEX /code MOD_CODE [/code NEW_MOD_CODE] [/type CLASS_TYPE] [/at DAY START_TIME END_TIME] [/venue VENUE]
Command properties:
Example:
Let’s say that the venue of the CS2103T lecture you just added changed to Home. You can edit the class by typing class edit 1 /code CS2103T /venue Home
. Mod Manager will direct you to the module’s page and it will reflect the updated venue as seen below.
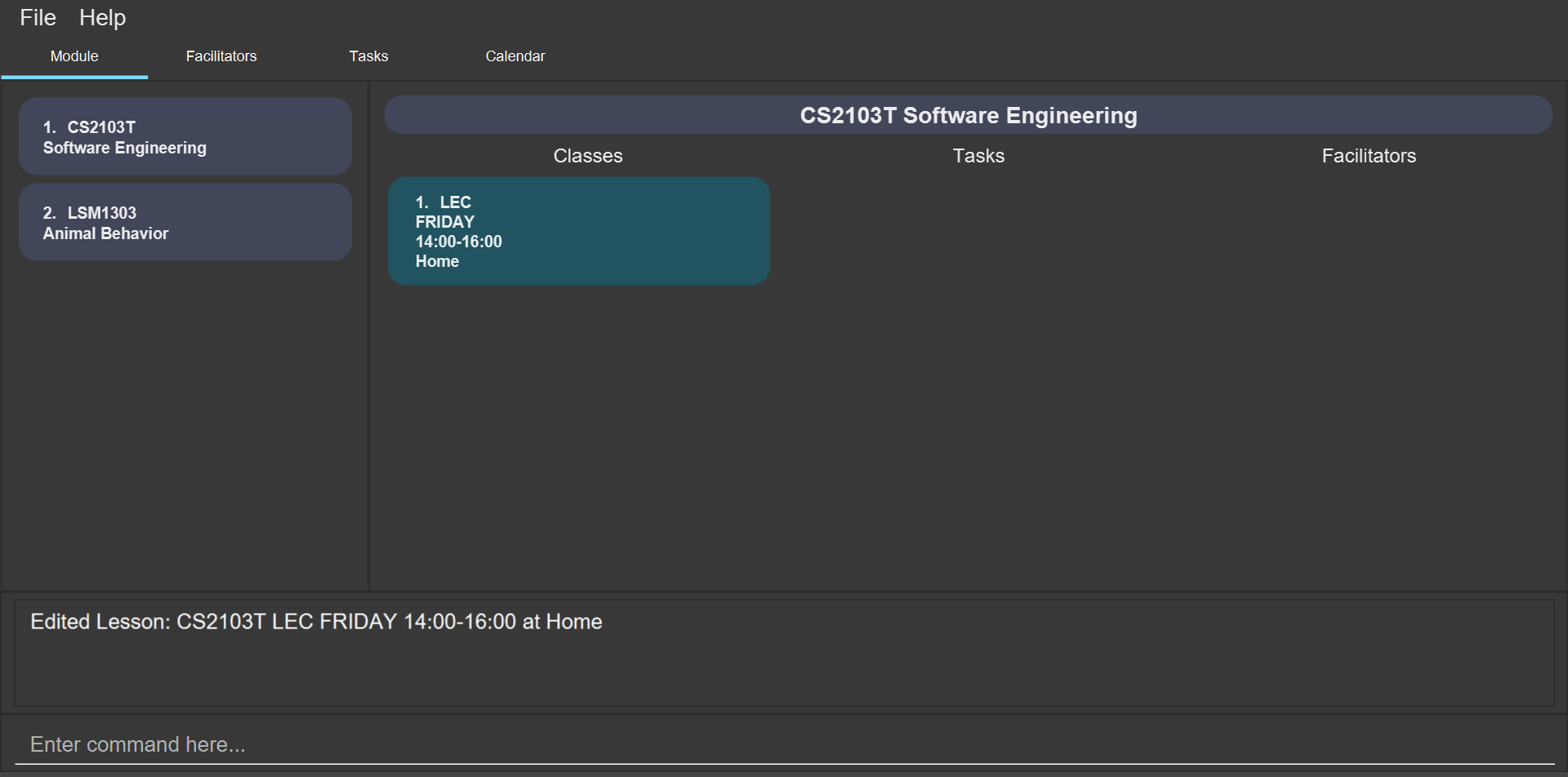
Deleting a class (Heidi)
You can delete the class from the module. The class to be deleted is selected by its index in the displayed module’s class list. You can view the module’s class list by using mod view MOD_CODE
as stated in section 3.2.3.
Format:
-
class delete INDEX /code MOD_CODE
Command properties:
Example:
You can delete the CS2103T lecture by typing class delete 1 /code CS2103T
. The class will not appear in the class list under the module CS2103T as seen below.
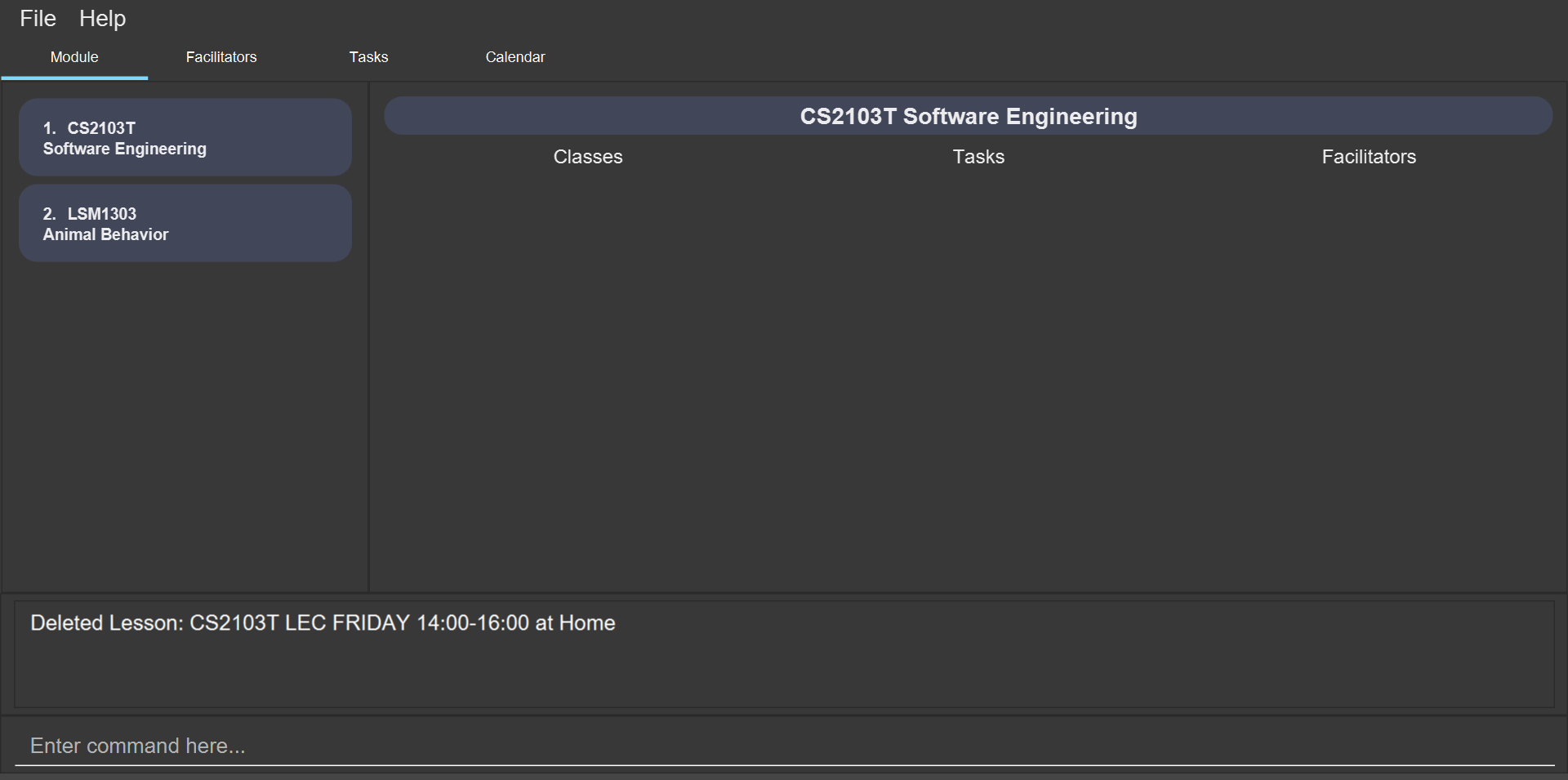
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Class Management Feature
The class feature manages the classes in Mod Manager and is represented by the Lesson
class.
A class has a ModuleCode
, LessonType
, DayAndTime
and venue
which is a String
.
It supports the following operations:
-
add
- Adds a class to Mod Manager. -
find
- Finds specific classes in Mod Manager. -
edit
- Edits a class in Mod Manager. -
delete
- Deletes a class in Mod Manager.
Implementation Details
Adding a class (Heidi)
The add class command allows user to add a class to Mod Manager. This feature is facilitated by LessonCommandParser
, LessonAddCommandParser
and LessonAddCommand
. The operation is exposed in the Model
interface as Model#addLesson()
.
Given below is an example usage scenario and how the lesson add mechanism behaves at each step.
-
The user executes the lesson add command and provides the module code, lesson type, day, start time, end time and venue of the lesson to be added.
-
LessonAddCommandParser
creates a newLesson
, then a newLessonAddCommand
. -
LogicManager
executes theLessonAddCommand
. -
ModManager
adds theLesson
toLessonList
.
The following sequence diagram shows how the lesson add command works:
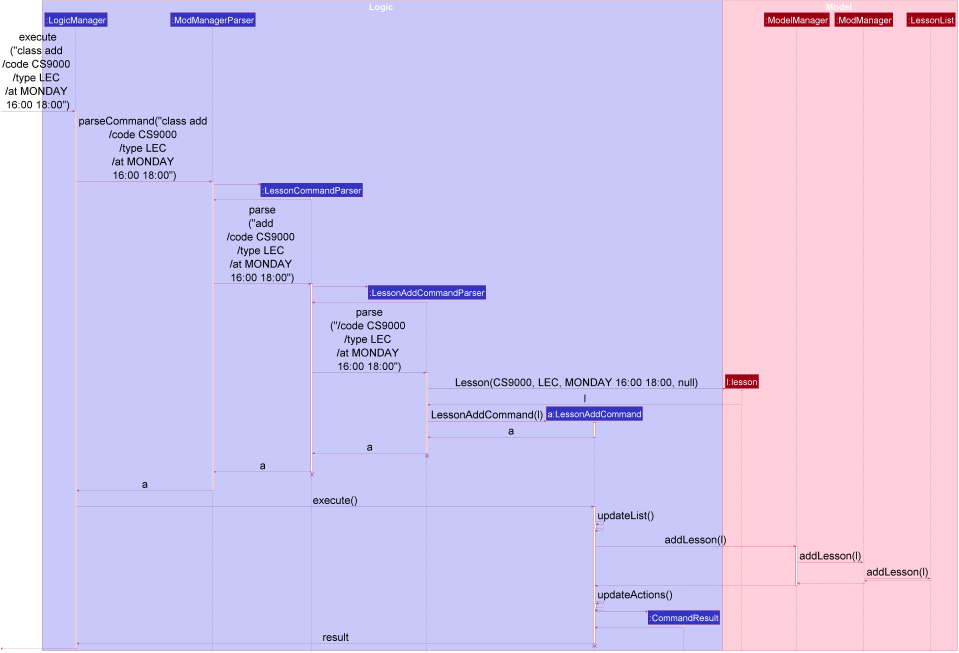
class add
Command
The lifeline for LessonCommandParser , LessonAddCommandParser and LessonAddCommand should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
The following activity diagram summarizes what happens when a user executes a lesson add command:
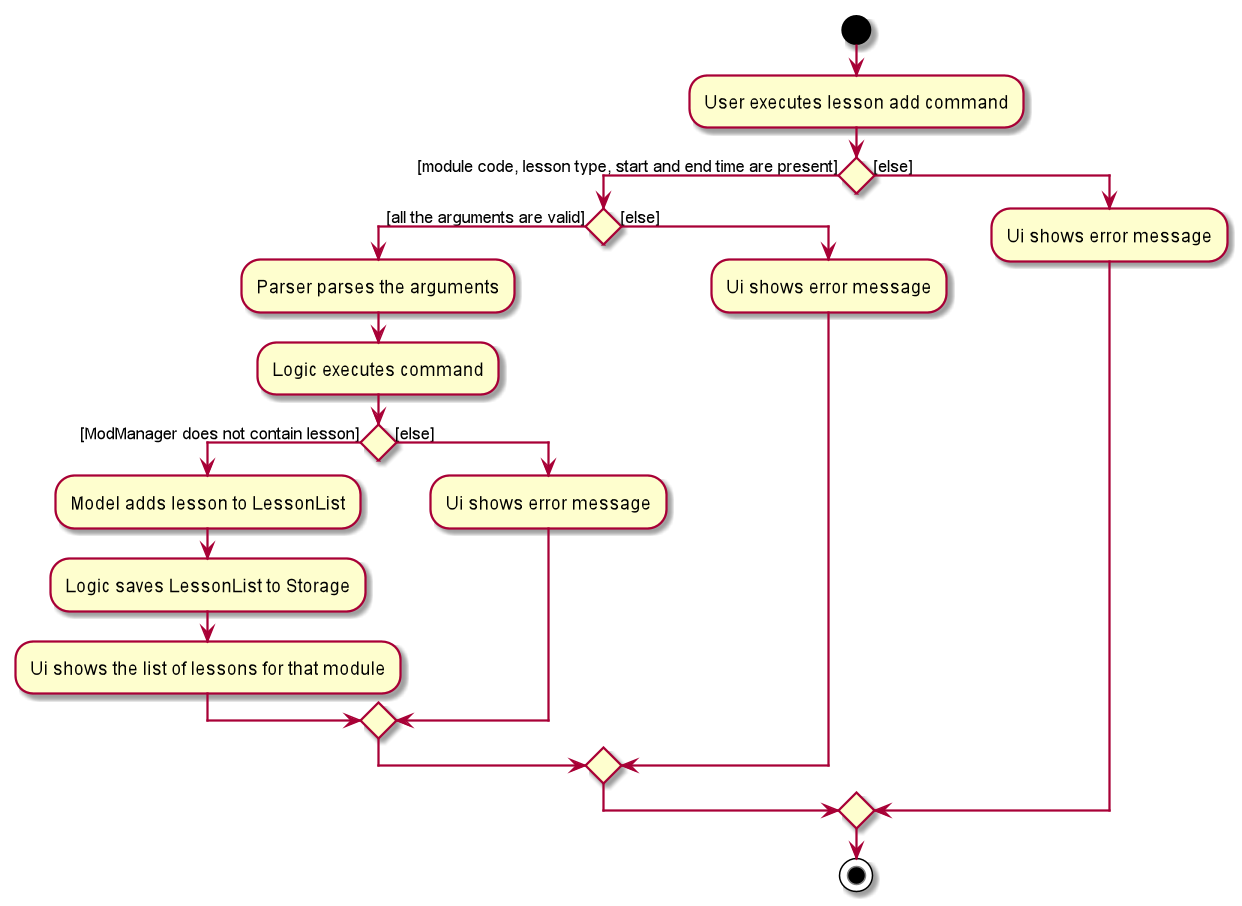
class add
CommandFinding a class (Heidi)
The find class command allows user to find a class to Mod Manager. This feature is facilitated by LessonCommandParser
, LessonFindCommandParser
and LessonFindCommand
. The operation is exposed in the Model
interface as Model#findNextLesson()
and Model#findLessonByDay
.
Given below is an example usage scenario and how the lesson find mechanism behaves at each step.
-
The user executes the lesson find command with the
next
prefix. -
LessonFindCommandParser
creates a newLessonFindCommand
. -
LogicManager
executes theLessonFindCommand
.
The following sequence diagram shows how the lesson find command works:
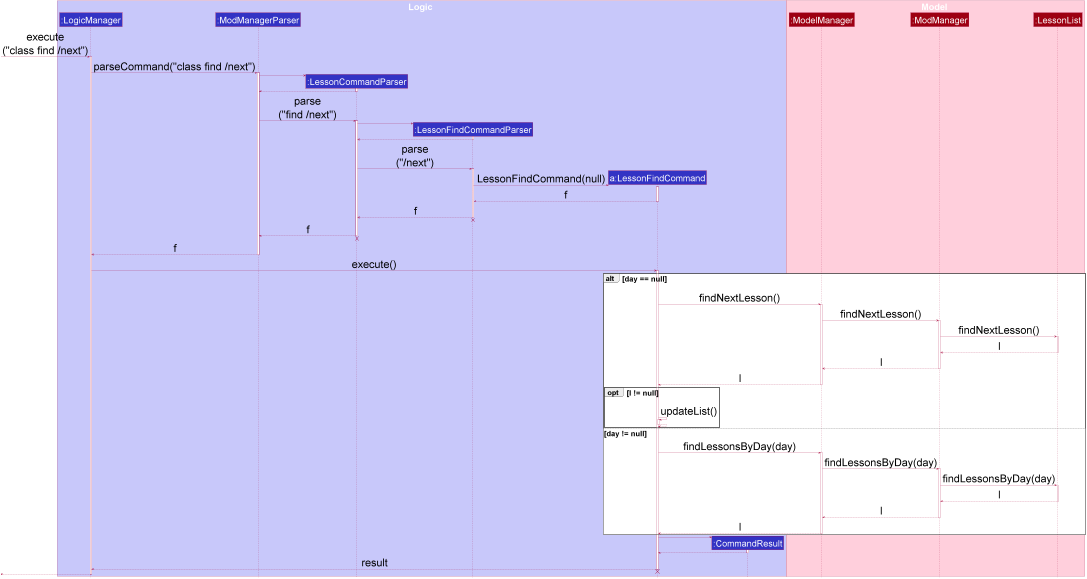
class find
Command
The lifeline for LessonCommandParser , LessonFindCommandParser , LessonFindCommand should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of the diagram.
|
The following activity diagram summarizes what happens when a user executes a lesson find command:
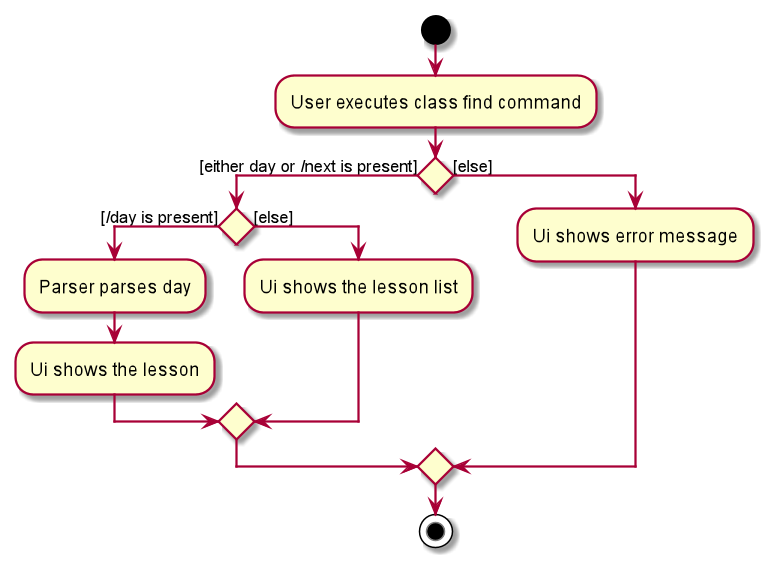
class find
CommandEditing a class (Heidi)
The edit class command allows user to edit a class to Mod Manager. This feature is facilitated by LessonCommandParser
, LessonEditCommandParser
and LessonEditCommand
. The operation is exposed in the Model
interface as Model#setLesson()
.
Given below is an example usage scenario and how the lesson edit mechanism behaves at each step.
-
The user executes the lesson edit command and provides the index of the lesson to be edited, the module code of the lesson and the fields to be edited.
-
LessonEditCommandParser
creates a newEditLessonDescriptor
with the fields to be edited. -
LessonEditCommandParser
creates a newLessonEditCommand
based on the index and module code, andEditLessonDescriptor
. -
LogicManager
executes theLessonEditCommand
. -
LessonEditCommand
retrieves thelesson
to be edited. -
LessonEditCommand
creates a newLesson
. -
ModManager
sets the existinglesson
to the newlesson
in theLessonList
.
The following sequence diagram shows how the lesson edit command works:
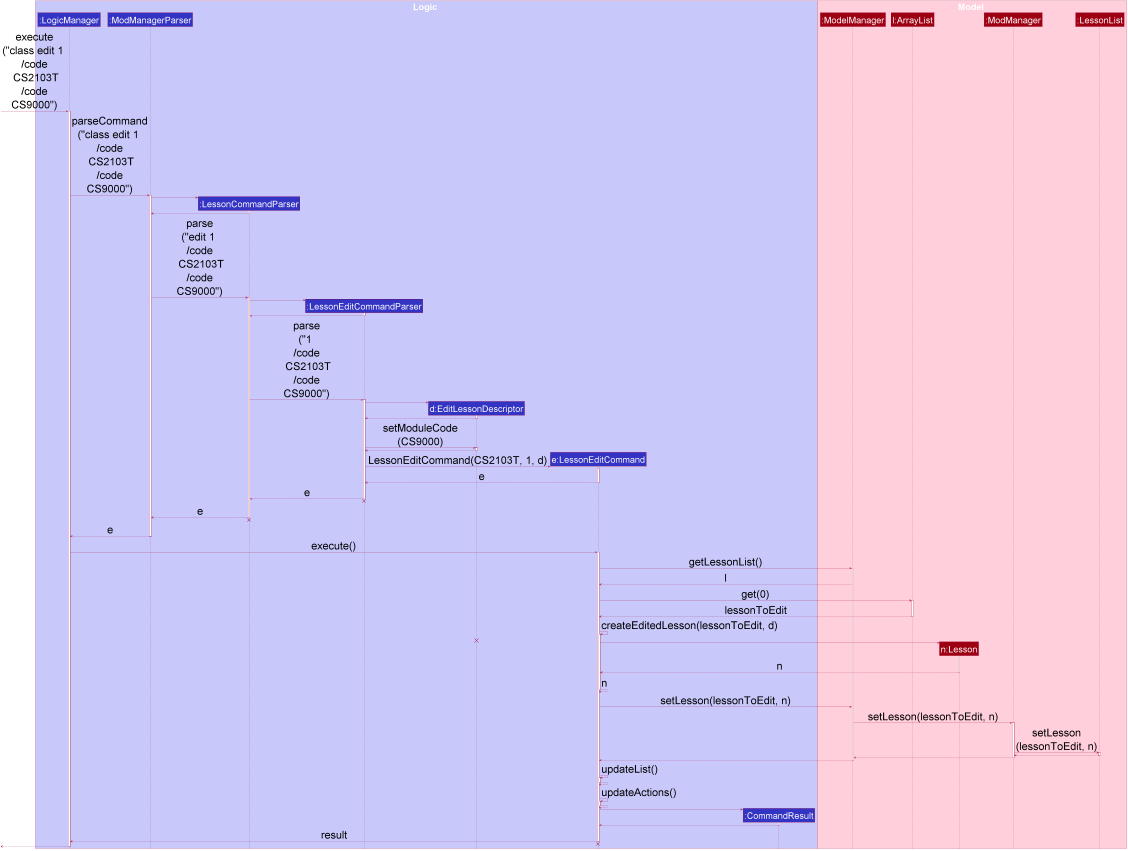
class edit
Command
The lifeline for LessonCommandParser , LessonEditCommandParser , EditLessonDescriptor and LessonEditCommand should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
The following activity diagram summarizes what happens when a user executes a lesson edit command:
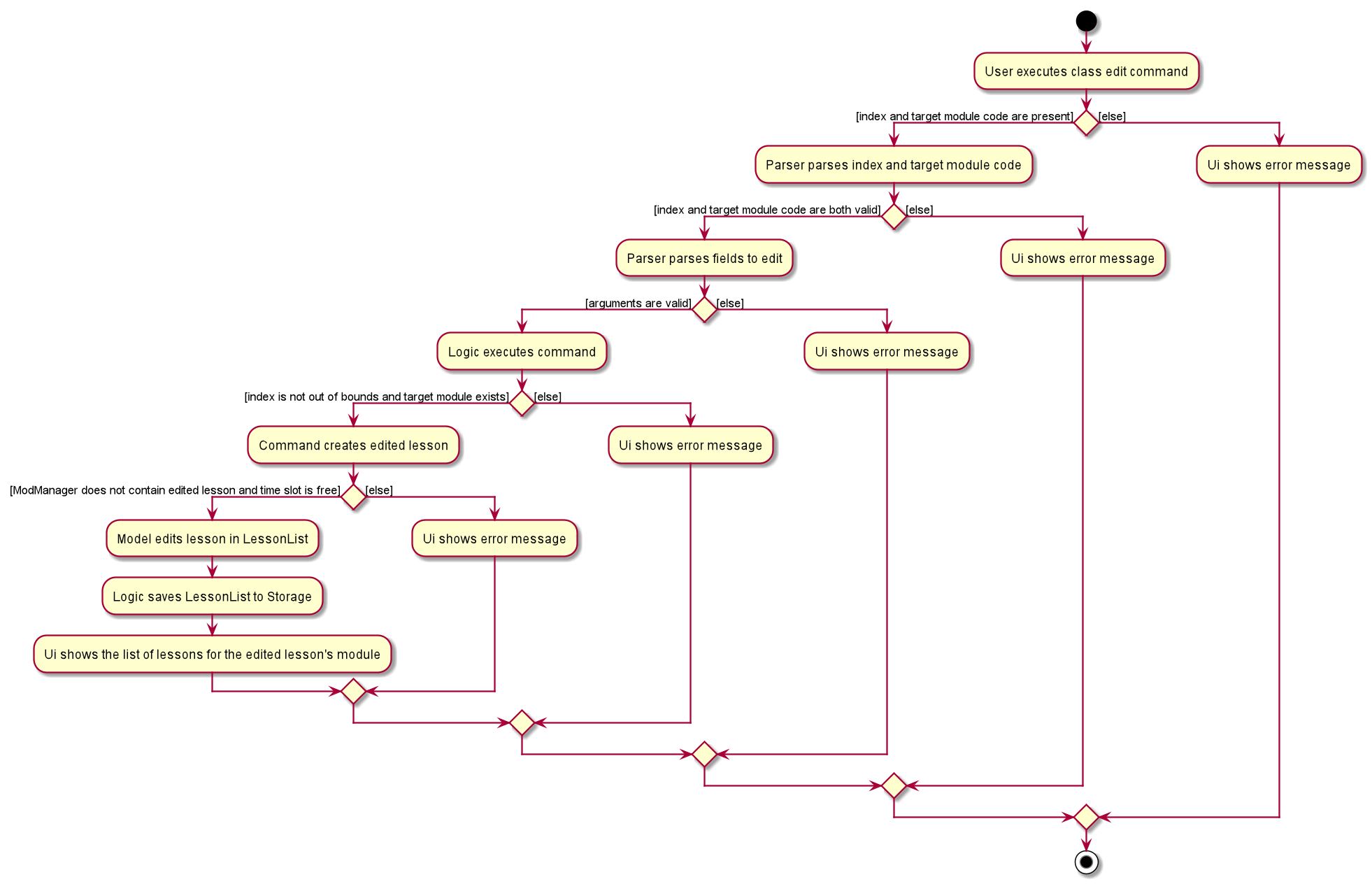
class edit
CommandDeleting a class (Heidi)
The delete class command allows user to add a class to Mod Manager. This feature is facilitated by LessonCommandParser
, LessonDeleteCommandParser
and LessonDeleteCommand
. The operation is exposed in the Model
interface as Model#removeLesson()
.
Given below is an example usage scenario and how the lesson delete mechanism behaves at each step.
-
The user executes the lesson delete command and provides the index of the lesson to be deleted.
-
LessonDeleteCommandParser
creates a newLessonDeleteCommand
. -
LogicManager
executes theLessonDeleteCommand
. -
LessonDeleteCommand
retrieves thelesson
to be deleted. -
ModManager
deletes theLesson
fromLessonList
.
The following sequence diagram shows how the lesson delete command works:
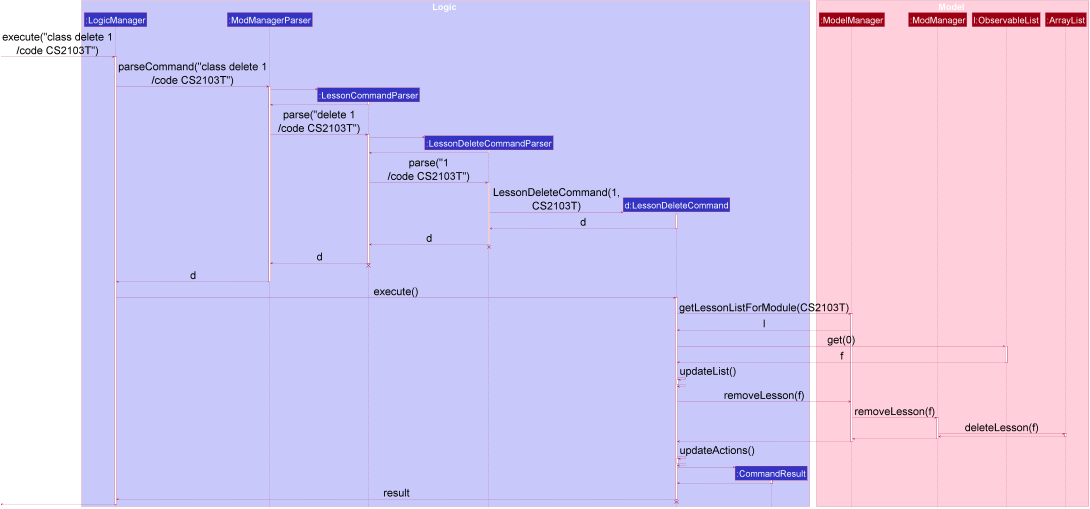
class delete
Command
The lifeline for LessonCommandParser , LessonDeleteCommandParser and LessonDeleteCommand should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of the diagram.
|
The following activity diagram summarizes what happens when a user executes a lesson delete command:
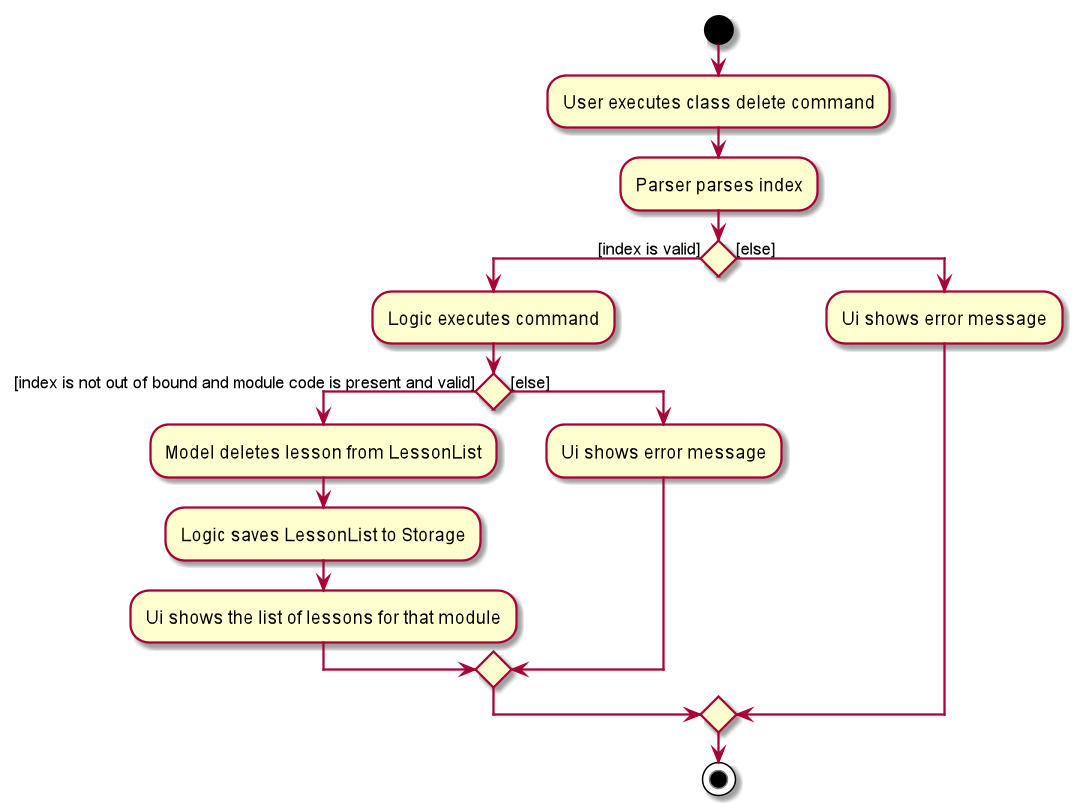
class delete
CommandDesign Considerations (Heidi)
Aspect: Prefix of day and time
-
Alternative 1 (current choice): Have one prefix for all three
day
,startTime
andendTime
fields.-
Pros: User types less.
-
Cons: When user wants to edit one field only, user have to key in other unnecessary details.
-
-
Alternative 2: Have one prefix each for
day
,startTime
andendTime
fields.-
Pros: Easier to parse and less invalid inputs to take note of. User can also edit any field.
-
Cons: More prefixes to remember and command will be very lengthy.
-
Appendix A: User Stories
Priorities:
High (must have) - * * *
Medium (nice to have) - * *
Low (unlikely to have) - *
Priority | As a … | I can … | so that … |
---|---|---|---|
|
new user |
see usage instructions |
I can refer to instructions when I forget how to use the App |
|
student |
add a module I am taking |
I can keep track of the information related to the module |
|
student |
add a class |
I can keep track of the classes I have for a particular module |
|
student |
add a task |
I can keep track of the tasks I have for a particular module |
|
student |
add facilitators' information |
I can keep track of the information of the facilitators |
|
student |
view information related to a module |
I can prepare for each module |
|
student |
view all tasks across all modules |
I can organise, plan, and manage my tasks better |
|
student |
view tasks for a specific module |
I can manage and keep track of homework, programming assignments, and other tasks specifically for the module |
|
student |
view all tasks not done |
I know what tasks are not yet completed and do them |
|
student |
view facilitators' information |
I can contact them when I need help |
|
student |
edit a module |
I can update the module |
|
student |
edit a class |
I can keep my classes up to date |
|
student |
edit a task |
I can keep my tasks up to date |
|
student |
mark a task as done |
I can keep track of what tasks I have completed and what I have not yet completed |
|
student |
edit a facilitator’s information |
I can keep their contact details up to date |
|
student |
delete a module |
I can use the App for different semesters |
|
student |
delete a class |
I can remove classes that I am no longer in |
|
student |
delete a task |
I can remove tasks that I no longer need to track |
|
student |
delete a facilitator’s information |
I can remove information that I no longer need |
|
busy student |
view schedule for the current week |
I can prepare for them |
|
busy student |
view schedule for the upcoming week |
I can prepare for them |
|
new user |
view all commands |
I can learn how to use them |
|
new user |
view commands for a specific feature |
I can learn how to use them |
|
user |
import and export data |
I can easily migrate the data to another computer |
|
user |
undo or redo my commands |
I can save time from trying to fix my mistakes |
|
student |
find a facilitator by name |
I can locate details of facilitators without having to go through the entire list |
|
student |
find tasks by description |
I can find the exact tasks that I want to do, such as a particular programming assignment on multimedia streaming |
|
student |
search tasks by its date |
I know what tasks are happening today, tomorrow, this month, next month, or any other time periods |
|
student |
find upcoming tasks |
I can prioritise them |
|
busy student |
find empty slots in my schedule |
I can manage my time easily |
|
user |
see my past commands quickly |
I can re-use a command without having to type it again |
|
student |
mark a task as done |
I can not take note of them anymore |
|
student |
add a priority level to a task |
I can prioritise my tasks |
|
student |
tag my tasks |
I can categorise them |
|
student |
see countdown timers |
I can be reminded of deadlines |
|
busy student |
I can receive reminders about deadlines and events the next day |
take note of them |
|
student |
mass delete the modules |
I can delete them quickly once the semester is over |
|
advanced user |
I can use shorter versions of a command |
type a command faster |
|
careless user |
undo my commands |
I can undo the mistakes in my command |
|
visual user |
see a clear GUI |
I can navigate the App more easily |
Appendix C: Use Cases
Use case: UC06 - Add class
-
1. User request to add a class and provides the details of the new class.
-
2. Mod Manager adds a class.
Use case ends.
-
1a. Compulsory fields are not provided or fields provided are invalid.
-
1a1. Mod Manager shows an error message.
Use case resumes from step 1.
-
Use case: UC07 - Find class by day
-
1. User request to list all the classes by day and provides the day.
-
2. Mod Manager replies with the list of classes.
Use case ends.
-
1a. Day provided is invalid.
-
1a1. Mod Manager shows an error message.
Use case resumes from step 1.
-
-
1b. No class on the day provided.
Use case ends.
Use case: UC08 - Find next class
-
1. User request to find the next class.
-
2. Mod Manager replies with the next class.
Use case ends.
-
1a. No next class.
Use case ends.
Use case: UC09 - Edit class
-
1. User request to edit a class and provides the index and necessary details to be edited.
-
2. Mod Manager edits the class.
Use case ends.
-
1a. Index is not provided or invalid, or details are not provided or invalid.
-
1a1. Mod Manager shows an error message.
Use case resumes from step 1.
-
Use case: UC10 - Delete class
-
1. User requests to delete a class and provides the index.
-
2. Mod Manager deletes the class.
Use case ends.
-
1a. Index is not provided or is invalid.
-
1a1. Mod Manager shows an error message.
Use case resumes from step 1.
-