Overview
This portfolio summarizes my contribution to Mod Manager, a software developed by a team of me and 4 other fellow CS2103T students. Over a period of 8 weeks, we have managed to morph Address Book Level 3 (AB3) into a CLI application that aims to assist students with managing information related to the modules they’re taking - classes, tasks, and facilitators. Mod Manager takes commands from users through a Command Line Interface (CLI), and displays information with a Graphic User Interface (GUI). It is written in Java, and has about 25 kLoc.
Below are my contributions to the project.
Summary of contributions
Major enhancements:
-
Added the ability to undo/redo previous commands
-
What it does:
-
Allows the user to undo previous commands that caused changes to the database.
-
Allows the user to reverse previous undo commands via the redo command.
-
-
Justification: This feature improves the product significantly because a user can make mistakes in commands and the app should provide a convenient way to rectify them.
-
Highlights: This enhancement affects almost all existing commands and any commands implemented in the future. Despite the challenge of having to understand the entire system, its implementation has been kept to be as convenient as possible, i.e. requiring very minor changes to other commands. A pattern has been constructed to help future commands integrate well with this enhancement.
-
-
Added the Task ID number system
-
What it does:
-
Allows the user to manage tasks across all modules without relying on visible lists.
-
-
Justification: Without a Task ID number, the user must always view the list of tasks before doing anything with a particular task. With this enhancement, a user can edit, delete, or mark as done a task anytime as long as they know its ID number.
-
Highlights: This enhancement imposes a visible constraint on the maximum number of tasks for each module, which can be helpful to understanding the system. There could have been many ways to implement the enhancement, but the current one was chosen due to its simpler nature and being easy to upgrade in the future.
-
Minor enhancements:
-
Added an internal command history that allows the user to navigate to previous commands using up/down keys.
-
Re-designed the calendar UI to the current version.
-
Added the ability to edit/delete modules, facilitators and tasks using module codes, facilitator names and task ID numbers respectively.
-
Refactored AB3 parser code to provide better abstraction, and be a pattern for other commands' implementations.
Code contributed:
Other contributions:
-
Project management:
-
Reviewed and merged pull requests on Github.
-
-
Documentation:
-
Community:
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Task feature
Editing a task (Nhat)
From here on, you will be introduced to Mod Manager’s task ID system.
You can edit a task’s description, its date and time details, or both by providing the correct task ID and the updated information.
Format:
-
task edit MOD_CODE ID_NUMBER [/desc DESCRIPTION] [/on DATE] [/at TIME]
Mod Manager will find the task associated with the module code and task ID number provided and update the information correspondingly.
The properties of DESCRIPTION
, DATE
and TIME
are described in the task add
command above.
Example: task edit CS2101 344 /desc OP2 /on 08/04/2020
Type the command into the command box. The task to edit in this example is marked by the red rectangle.
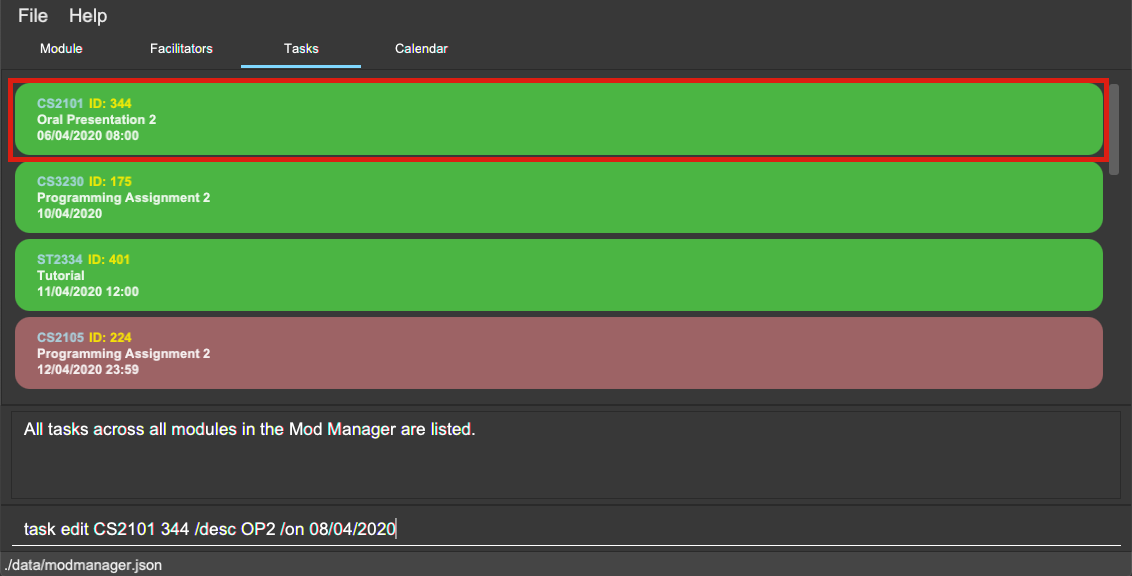
task edit
After pressing Enter, you will see that the task has been edited.
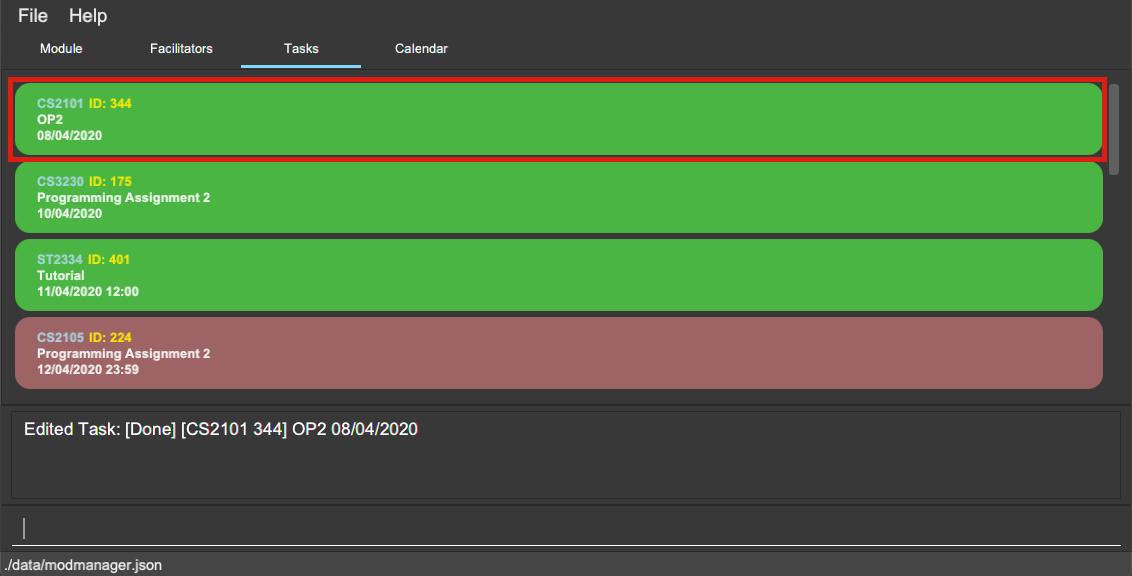
task edit
Since you may want to remove the date and time of a task, Mod Manager provides you a way to do so. Note that if you try to remove the date and time from a task without these values,
Format:
-
task edit MOD_CODE ID_NUMBER [/desc DESCRIPTION] /on non
Example: task edit ST2334 401 /on non
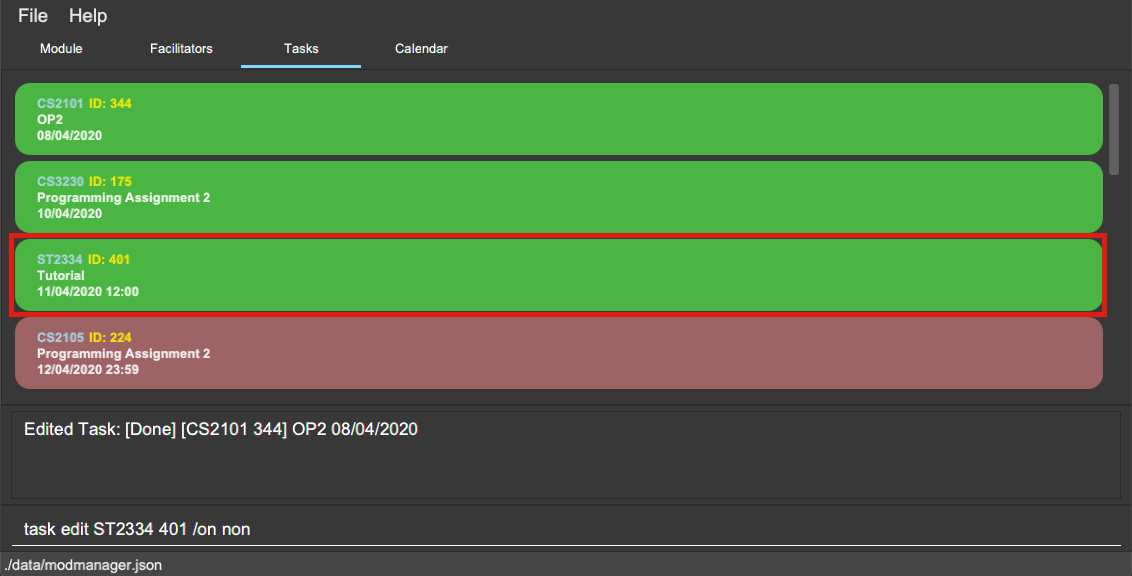
Again, press Enter and you will see its date and time has been removed. Its order in the list may be changed due to the sorting property of the task list.
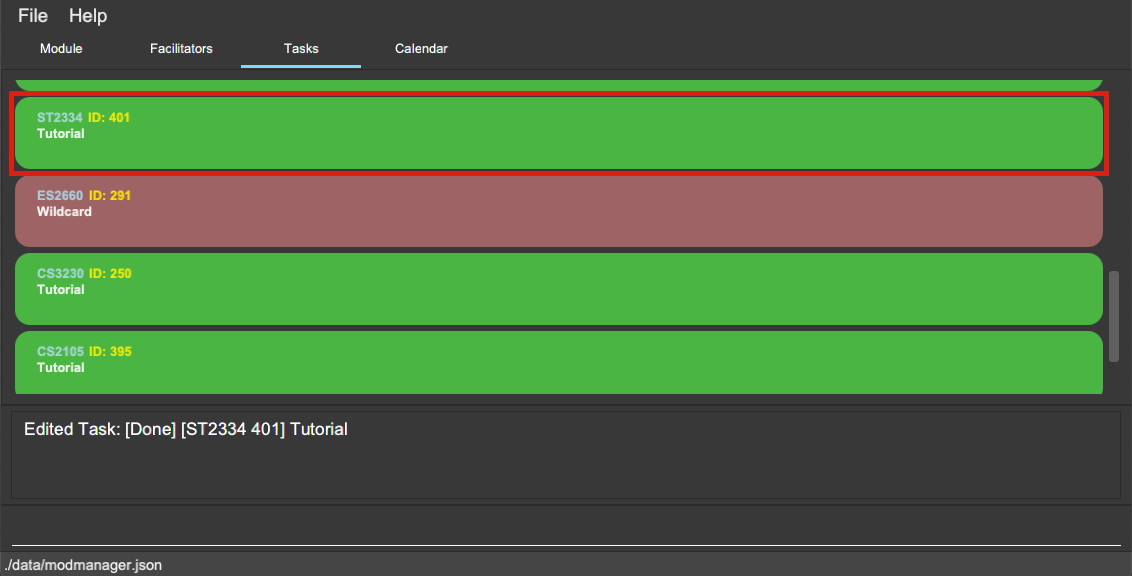
Deleting a task (Nhat)
You can delete a task from Mod Manager’s system.
Format:
-
task delete MOD_CODE ID_NUMBER
Example: task delete CS2101 344
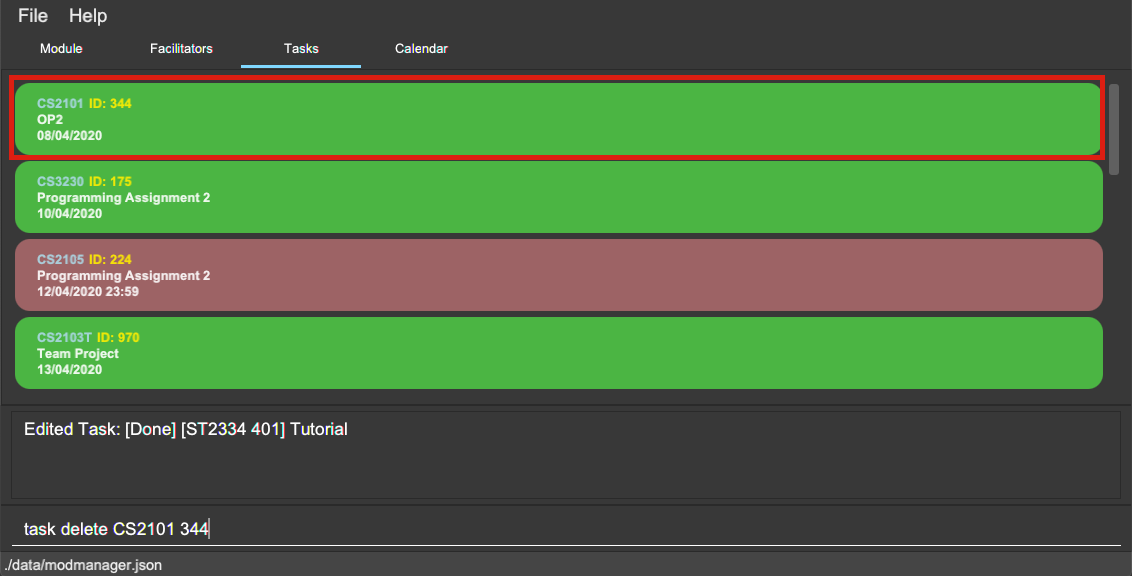
Press Enter and the task will be gone from the list.
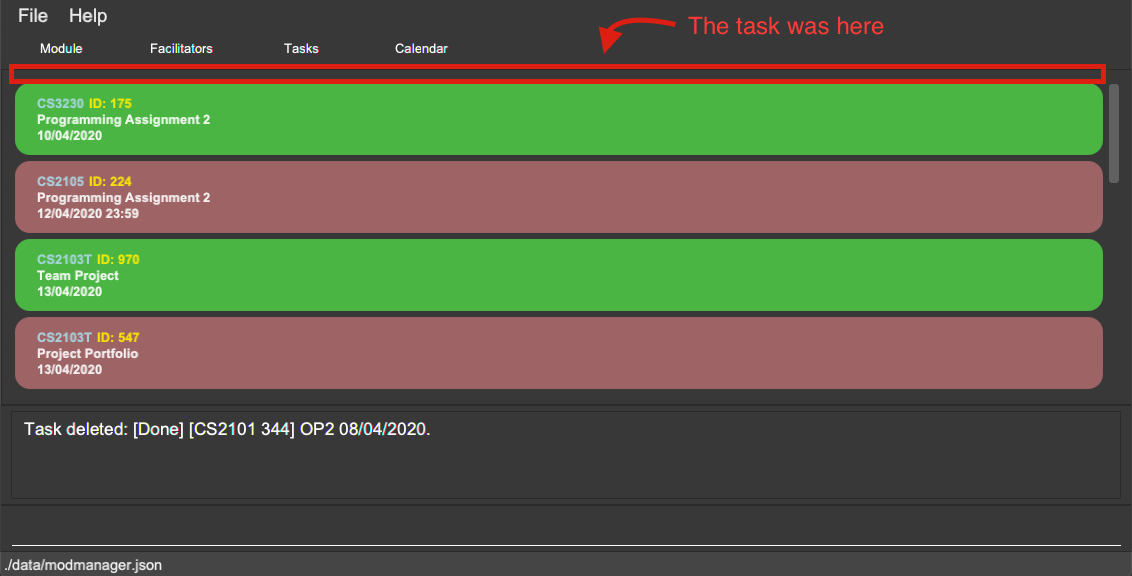
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Model Component
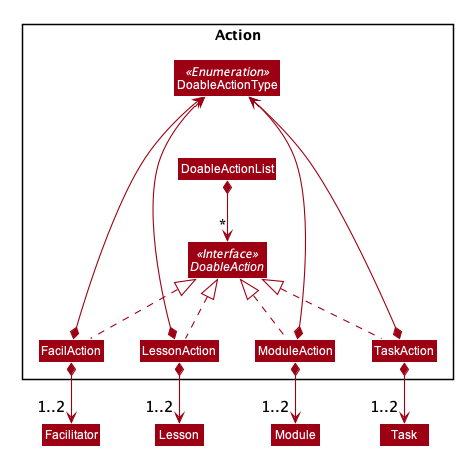
-
DoableAction
is an interface of actions that can be undone/redone in Mod Manager, stored in theAction
package. -
Actual implementations of
DoableAction
areModuleAction
,LessonAction
,FacilAction
, andTaskAction
, each of which has aDoableActionType
value to assist how the undo/redo process is carried out. -
A
DoableActionList
comprises of instances of classes mentioned above.
General Features
Undo/Redo command (Nhat)
Each add/edit/delete action is captured as a DoableAction
. Every time a DoableAction
is performed, it will be recorded
to the DoableActionList
. Thus, after each add/edit/delete command execution, a suitable DoableAction
will be be
created and recorded. Other sections might not mention this again.
DoableActionList
stores two Stacks
of DoableAction
called primary
and secondary
.
The mechanism of undo
is given below. For redo
, it is exactly the same.
-
The user executes the
undo
command. TheUndoCommandParser
creates anUndoCommand
. -
LogicManager
executes theUndoCommand
. -
ModelManager
callsundo
method ofDoableActionList
to reverse the effect of the most previousDoableAction
.
Navigating through past commands with up/down keys (Nhat)
This feature applies to each usage session. The mechanism is below.
-
Each time the user types anything and presses Enter in the
CommandBox
, the input will be saved to theUserInputHistory
. -
When an
up
key is pressed, the latest previous input will be retrieved fromUserInputHistory
and display at theCommandBox
. If there are no previous inputs to show, theCommandBox
will either stay the same or become empty. -
When a
down
key is pressed, the most previously input seen by pressingup
will be shown at theCommandBox
. When there are no inputs to show, theCommandBox
will become empty.
Clear command
This command simply clears all the data from the system. The action is not undo-able. Its mechanism is simple.
-
The user inputs a clear command.
-
ModelManager
will replace the currentModManager
instance with a newly created one that doesn’t contain any data.
Help command
This command will pop up a window showing a link to the User Guide. The user simply inputs a help command and the window will appear.
Design Considerations (Nhat)
Aspect: Undo/Redo Implementation
-
Alternative 1: Saves the entire database every time an add/edit/delete action occurs.
-
Pros: Easy to implement.
-
Cons: High memory consumption during a usage session, and potentially causing lag if the database is huge.
-
-
Alternative 2 (current choice): Each feature that involves adding/editing/deleting data to the database would have a corresponding class extending
DoableAction
. For example, Module Management feature would have aModuleAction
class that extendsDoableAction
. This special class will contain specific details on how to revert the effect of each add/edit/delete action.-
Pros: Low memory consumption during a usage session, leading to potentially more consistent performance.
-
Cons: Difficult to implement.
-
Alternative 2 was chosen as it could provide better performance with a huge database, and partly because our team enjoyed some extra challenge.
Task Management Feature
Editing a task (Nhat)
The task edit
command allows user to edit a task in Mod Manager.
The fields that can be edited are: description, and task date and time.
This feature is facilitated by TaskCommandParser
, TaskEditCommandParser
and TaskEditCommand
.
The operation is exposed in the Model
interface as Model#setTask()
.
Given below is an example usage scenario and how the task edit
mechanism behaves at each step.
-
The user executes the task edit command and provides the
moduleCode
and thetaskNum
of the task to edit, and the fields to be edited. -
TaskEditCommandParser
creates a newEditTaskDescriptor
with the fields to be edited. -
TaskEditCommandParser
creates a newTaskEditCommand
based on themoduleCode
andtaskNum
, andEditTaskDescriptor
. -
LogicManager
executes theTaskEditCommand
. -
TaskEditCommand
retrieves themoduleCode
andtaskNum
of thetask
to be edited, and then retrieves the actualtask
fromModManager
. -
TaskEditCommand
creates a newTask
. Since the user can usetask edit
to remove a task’s date/time, a specialTaskDateTime
has been set to01/01/1970
to help with theedit
command. Essentially, if theEditTaskDescription
carries such date, the newly createdTask
will not have aTaskDateTime
and be of typeNonScheduledTask
. An assumption about user inputs is made here: no one will actually input01/01/1970
as a date. -
ModManager
sets the existingtask
to the newtask
in theUniqueTaskList
. -
The
edit
action is recorded inModelManager
.
The following sequence diagram shows how a TaskEditCommand
is created after the parsing steps:
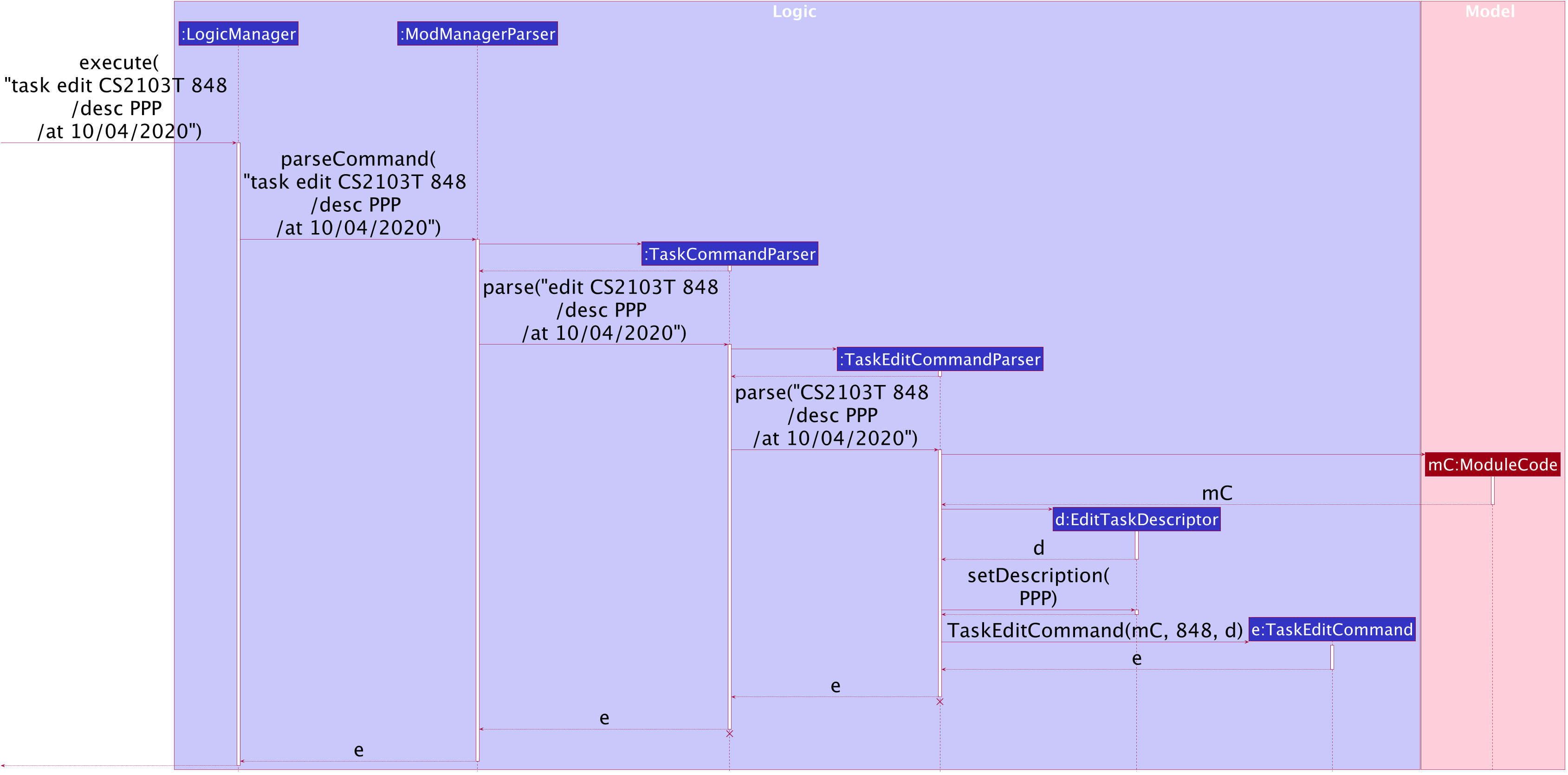
TaskEditCommand
Creation Steps
The lifeline for TaskCommandParser and TaskEditCommandParser should end at
the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
|
The execution of a TaskEditCommand
is described below.
-
List<Task> current
is retrieved by callingmodel.getFilteredTaskList
. -
The correct
taskToEdit
is retrieved fromcurrent
by turning it into astream
and use thereduce
method. -
The
editedTask
is created using methodcreateEditedTask
. -
model
setstaskToEdit
toeditedTask
in theUniqueTaskList
via calls toModManager
. -
An
editTaskAction
is created and added tomodel
. -
A
CommandResult
is returned.